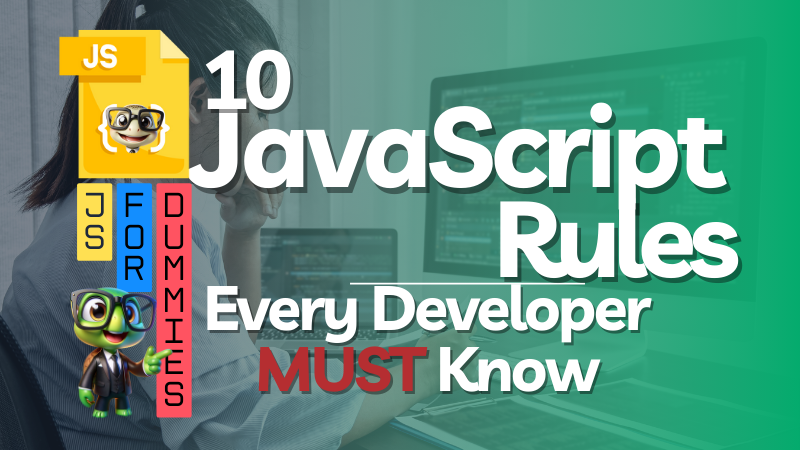
Discover essential JavaScript programming rules that every developer must follow to write clean, efficient, and error-free code. Improve your skills with these best practices and tips.
Table of Contents
- Introduction
- 1. Always Use JS Code Inside
- 2. The
- 3. You Can Use the
- 4. To Generate Output, Use document.write(), console.log(), window.alert(), and innerHTML
- 5. Always Comment Your Code Using // or /* */
- 6. Declare Variables with let, const, and var
- 7. JavaScript Will Generate Errors and Show Them in the Console
- 8. Always Avoid Global Variables
- 9. Always Use Semicolons to Terminate Statements
- 10. Keep Your Code DRY (Don’t Repeat Yourself)
- Conclusion
- Frequently Asked Questions (FAQs)
Introduction
JavaScript is the backbone of modern web development, powering everything from interactive web pages to complex applications. Whether you are a beginner or an experienced developer, following certain rules can help you write more efficient and maintainable code. In this article, we will delve into the 10 most important JavaScript programming rules that every developer must know. These rules not only help you avoid errors but also ensure that your code adheres to best practices. Let’s get started!
1. Always Use JS Code Inside <script>
Tag
One of the most fundamental rules when writing JavaScript is ensuring that your code is placed inside a <script>
tag. JavaScript cannot run without this tag, as it tells the browser that the enclosed code is JavaScript.
Why Is It Important?
- The
<script>
tag is a way to embed or link JavaScript into HTML. - Without it, the browser will not recognize your code and won’t execute it.
Example:
<script>
console.log("Hello, World!");
</script>
By using this tag, you’re ensuring that your JavaScript is recognized and executed correctly.
2. The <script>
Tag Can Be Used Anywhere in HTML
The flexibility of JavaScript is one of the reasons it’s so popular. The <script>
tag can be placed anywhere within your HTML document, but its location matters.
Where Should You Place Your Scripts?
- Head Section: If you place your script inside the
<head>
tag, it will load before any HTML elements are rendered. This can sometimes cause issues with DOM manipulation. - Body Section: Placing scripts at the end of the body is the recommended approach. This ensures that all the HTML elements are loaded before the script is executed.
Example:
<!-- In the Head -->
<head>
<script src="script.js"></script>
</head>
<!-- At the End of Body -->
<body>
<script src="script.js"></script>
</body>
Best Practice:
Always place your JavaScript at the bottom of your HTML document before the closing </body>
tag to avoid render-blocking.
3. You Can Use the <script>
Tag Multiple Times
There is no limit to how many <script>
tags you can include in an HTML document. You can have multiple scripts throughout the page, and this can help keep your code modular and organized.
Why Is This Useful?
- You can separate different parts of your code into smaller files.
- It’s easier to debug and manage multiple smaller files instead of one large script.
Example:
<script src="script1.js"> </script>
<script src="script2.js"> </script>
Tip:
Use the async
or defer
attributes for external scripts to prevent them from blocking the rendering of the page.
4. To Generate Output, Use document.write()
, console.log()
, window.alert()
, and innerHTML
There are various methods available in JavaScript for displaying output. Each method serves a different purpose, and understanding when to use them is crucial.
document.write()
: This method is used to write directly to the HTML document. However, it is generally not recommended for modern development because it can overwrite the entire document if used incorrectly.
Example:
document.write("Hello to All");
console.log()
: Used for debugging, this method outputs information to the browser’s console.
Example:
console.log("This is output to the console.");
window.alert()
: This displays a pop-up alert message to the user. It’s useful for quick notifications.
Example:
codewindow.alert("This is an alert message.");
document.getElementById("demo").innerHTML
: This method manipulates the DOM, allowing you to change the content of an element.
Example:
codedocument.getElementById("demo").innerHTML = "<h2>This is the inner HTML of a paragraph.</h2>";
Best Practice:
Use console.log()
for debugging and avoid document.write()
in production code, as it can cause performance issues.
5. Always Comment Your Code Using //
or /* */
Comments are an essential part of writing clean and understandable code. They help others (and your future self) understand what the code does.
Types of Comments:
- Single-line comments: Use
//
for comments on a single line.
Example:
// This is a single-line comment
let x = 5;
- Multi-line comments: Use
/* */
for comments spanning multiple lines.
Example:
/*
This is a multi-line comment
which can span across multiple lines.
*/
let y = 10;
Why Comment?
- It improves code readability.
- It helps with debugging and collaboration.
- It explains complex logic.
6. Declare Variables with let
, const
, and var
JavaScript provides three primary ways to declare variables: let
, const
, and var
. It’s important to know when to use each one.
let
: Uselet
when the value of a variable can change. It provides block-scoping, making it the preferred choice for most variables.const
: Useconst
for variables whose values should not change. This ensures immutability and makes your code more predictable.var
:var
is function-scoped and is considered outdated for modern JavaScript. It’s better to avoid usingvar
in favor oflet
andconst
.
Example:
javascriptCopy codelet name = "John"; // Use let for variables that will change
const pi = 3.14; // Use const for constants
Best Practice:
- Use
let
andconst
instead ofvar
to avoid scope issues. - Always prefer
const
for variables that won’t change.
7. JavaScript Will Generate Errors and Show Them in the Console
One of JavaScript’s key features is its ability to catch errors and display them in the browser’s developer console. Errors can range from syntax mistakes to logical flaws in your code.
How to Handle Errors:
- Open the developer tools by pressing
Ctrl+Shift+I
orF12
(depending on the browser). - Look under the “Console” tab to view errors.
- Read the error message to understand what went wrong.
Example of an error:
let x = 5;
console.log(y); // ReferenceError: y is not defined
Tip:
Use try...catch
blocks to handle errors gracefully and provide custom error messages.
8. Always Avoid Global Variables
Global variables can cause unpredictable behavior and conflicts between different parts of your code. They are accessible from anywhere in the code, which increases the risk of accidental overwrites.
How to Avoid Global Variables:
- Declare variables using
let
orconst
inside functions or blocks. - Use IIFE (Immediately Invoked Function Expressions) to contain variables within a local scope.
Example:
(function() {
let localVar = "I'm local!";
console.log(localVar);
})();
9. Always Use Semicolons to Terminate Statements
Although JavaScript allows optional semicolons due to Automatic Semicolon Insertion (ASI), it’s a best practice to always explicitly end statements with semicolons.
Why Semicolons Matter:
- They prevent potential errors caused by ASI.
- They ensure your code behaves as expected.
Example:
let x = 10;
x++;
10. Keep Your Code DRY (Don’t Repeat Yourself)
One of the most crucial principles in programming is to avoid repetition. Keeping your code DRY ensures that you don’t write redundant code and helps maintain consistency.
How to Implement DRY:
- Use functions to group repetitive code.
- Reuse components and logic wherever possible.
Example:
function greet(name) {
return `Hello, ${name}!`;
}
console.log(greet("Alice"));
console.log(greet("Bob"));
Conclusion
By following these essential JavaScript rules, you’ll write cleaner, more maintainable, and error-free code. Whether you are just starting or you’ve been programming for years, adhering to best practices like using proper tags, commenting your code, handling errors, and avoiding repetition will take your coding skills to the next level.
Frequently Asked Questions (FAQs)
1. Why is it important to use const
instead of let
?
const
ensures that the value of a variable cannot be changed once it’s set, making the code more predictable and reducing bugs.
2. Can I put JavaScript inside a <div>
tag?
No, JavaScript code must be placed inside a <script>
tag. However, you can use JavaScript to modify the contents of a <div>
.
3. What are some common JavaScript errors and how can I debug them?
Common errors include syntax errors, undefined variables, and logic errors. Use console.log()
and the browser’s developer tools to debug them.
4. Is it okay to use var
in modern JavaScript?
While var
still works, it is outdated and can cause scope issues. It’s better to use let
and const
in modern JavaScript.