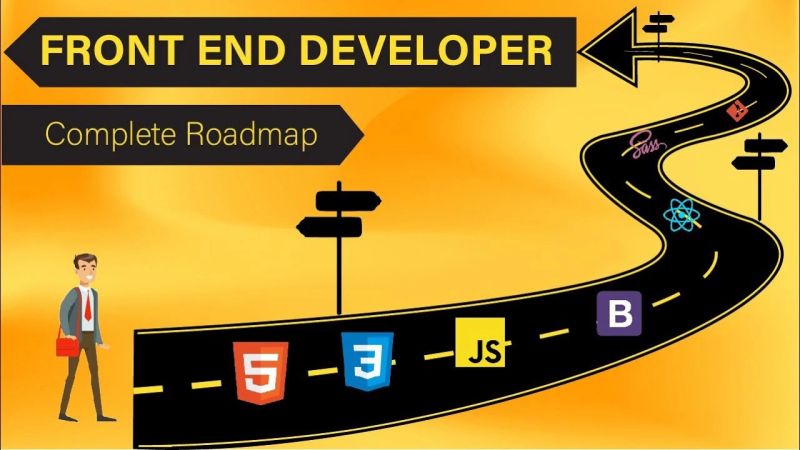
Complete FullStack Developer Roadmap
Unlock your full potential as a developer with our Complete FullStack Developer Roadmap! This comprehensive guide takes you through every essential step to become a proficient full-stack developer. Whether you’re just starting or looking to expand your skills, this roadmap provides a clear, structured path to mastering front-end and back-end development, databases, version control, deployment, and more. Dive into real-world tips, tool recommendations, and best practices that will help you build scalable, dynamic applications from scratch. Start your journey to becoming a full-stack expert today!
Introduction:
In today’s tech-driven world, full-stack development has become one of the most sought-after skills. But with so much to learn, where do you start? This blog is your ultimate guide to navigating the Complete FullStack Developer Roadmap. From understanding the basics of web development to mastering advanced concepts, we’ll walk you through the technologies, frameworks, and tools you need to know. Whether you’re a beginner or an experienced developer aiming to upskill, this roadmap will help you build a strong foundation and achieve your goals efficiently. Let’s embark on this exciting journey to becoming a full-stack developer!
Complete FullStack Developer Roadmap
Here are 9 steps that you need to follow in order to become a successful Fullstack Web developer. [ With Resources To Learn + Jobs]
Step 1 → Learn HTML CSS and JS
Step 2 → Learn Git and GitHub
Step 3 → Learn Frontend Frameworks [React Recommended]
Step 4 → Learn Nodejs – Python
Step 5 → Learn Backend Frameworks
Step 6 → Learn Database
Step 7 → Build Full Stack Projects
Step 8 → Host Your Project [AWS]
Step 9 → Apply for Jobs
[Build Projects After Each Step]
1. Learn HTML, CSS, and JavaScript
HTML
– Basic syntax and rules
– Semantic HTML
– Forms and validations
– Embedding media
– Accessibility standards
CSS
– Basic styling properties (color, font-size, background, etc.)
– Box model
– Positioning and display properties
– CSS Grid and Flexbox
– Responsive design and Media Queries
– CSS Variables
– Transitions and Animations
JavaScript
– Basics (variables, data types, operators, functions, loops, conditionals)
– ES6 features (let & const, arrow functions, classes, modules, destructuring, template strings)
– Asynchronous JS (Promises, async/await)
– Fetch API / AJAX
– JSON
– Error handling and debugging
– DOM Manipulation
– Event handling
2. Learn Git and GitHub
Git
– Setting up a repository
– Git lifecycle (add, commit, push, pull)
– Branching and merging
– Handling merge conflicts
– Git log
GitHub
– Creating a repository
– Pull requests
– Forking a repository
– GitHub Pages for hosting
3. Learn Frontend Framework/Library
[Learn any one]
React.js
– JSX
– Components (Functional and Class)
– State and Props
– Lifecycle methods
– Hooks
– Routing with react-router-dom
– Context API and Redux for state management
Vue.js
– Vue instance and Vue CLI
– Vue components and directives
– Vue Router
– Vuex for state management
Angular
– TypeScript basics
– Components and Modules
– Services and Dependency Injection
– Routing
– Forms
– HTTPClient
– RxJS and Observables
4. Advanced JavaScript & TypeScript
– Understanding prototypes and prototypical inheritance
– Deep dive into ‘this’ keyword
– Closures
– Webpack and Babel
– Design patterns in JavaScript
– Basics of TypeScript
5. Learn Backend Language/Framework
Node.js/Express
– Understanding the event loop
– Building a server with Express.js
– Handling different types of requests (GET, POST, PUT, DELETE)
– Middleware
– Error handling
– Authentication and Authorization
Python/Django/Flask
– Python basics
– Setting up a server with Flask/Django
– Models and migrations
– Django/Flask views and templates
– Django/Flask forms
– User Authentication
6. Learn Database Technology
SQL (MySQL, PostgreSQL, etc.)
– Basic SQL commands (SELECT, INSERT, UPDATE, DELETE)
– Joins
– Normalization
– Indexing and query optimization
NoSQL (MongoDB)
– Document databases
– Collections and documents
– Basic CRUD operations
7. Learn Authentication
– Sessions and Cookies
– JSON Web Tokens (JWT)
– OAuth 2.0
– Passport.js (if using Node.js)
8. Learn Caching Strategies
– Importance of caching
– In-memory databases (Redis)
– Browser caching
9. Learn about Testing [optional]
– Unit Testing
– Integration Testing
– End-to-End Testing
– Tools: Jest, Mocha, Chai, etc.
10. Understand DevOps Principles
– Introduction to Docker and Kubernetes
– Continuous Integration and Continuous Deployment (CI/CD)
– Understanding cloud platforms (AWS, Azure, Google Cloud)
11. Build Full Stack Projects
– Idea generation and selection
– Design and prototyping
– Implementing frontend and backend
– Database design and integration
– Testing
– Deployment
12. Host Your Project
– Introduction to cloud hosting platforms
– Configuring and deploying applications
– Setting up a custom domain
– Enforcing HTTPS
– Continuous deployment from GitHub
13. Learn about Microservices and Serverless Architecture (optional)
– Understanding the concept of microservices
– Serverless computing basics
– AWS Lambda, Google Cloud Functions, or Azure Functions
14. Apply for Jobs
[This shouldn’t be step 14, you should always look for opportunities and make connections!]
– Resume building
– Technical interview preparation
– LeetCode, HackerRank, and other coding challenges
– Networking on LinkedIn and Twitter
Resources You Can Use
– FreeCodeCamp [Youtube] [FREE] you can find all the tutorials you need to learn fullstack
– Udemy [Paid] You can follow courses on Udemy to build projects. [Code Along can be a great practice]
– Hashnode & Dev .to [FREE] Read and Write Blogs on the technologies.
– Also you take 100DaysOfCode challenge and share your progress here.