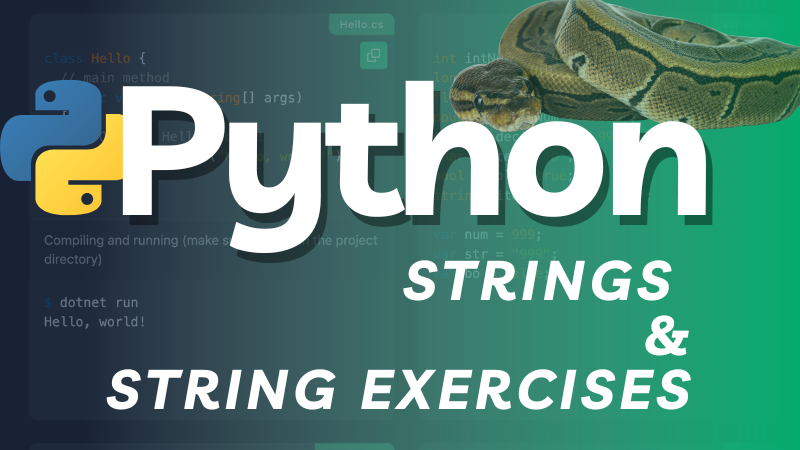
Python String Tutorial and String Coding Exercise
In Python, a string is a sequence of characters enclosed in quotes. Strings are used to handle text, and Python provides several methods to manipulate and operate on these text-based sequences efficiently. Strings in Python are immutable, meaning they cannot be changed after they are created, but various string methods allow us to create modified versions of the original string.
Table of Content
- Creating Strings in Python
- Single and Double Quotes
- Multi-Line String with Trible Quotes
- Why Use Triple Quotes?
- String Methods in Python
- Python String Indexing and Slicing
- Examples of Python String Methods
- Real World Python String Tasks
- Task 1: Replacing Characters Without using the replace()
- Task 2: Extract and Format Telephone Number using Python
- Task 3: Extract Information from a String using Python
- Task 4: Remove Spaces from a String using Python
- Task 5: Convert 2-Digit Year to 4-Digit Year using Python
- Task 6: Format Name
- Task 7: Convert Number to Words
- Task 8: Date Conversion to Month Format
- Additional Real-World Python String Tasks
Creating Strings in Python
Single and Double Quotes
In Python, strings can be created using either single (‘ ‘) or double quotes (” “). Both work the same way, so you can use whichever you prefer. This is useful if you want to include one type of quote inside the string without needing escape characters.
# Using single or double quotes
string1 = 'Hello'
string2 = "World"
# Multi-line string using triple quotes
multi_line = '''This is a
multi-line string.'''
string1 = ‘Hello’: Here, we’re defining a string and storing it in a variable called string1. Since we used single quotes, the string is defined as ‘Hello’.
string2 = “World”: Similarly, we’re creating another string but with double quotes, “World”, and storing it in string2.
Both string1 and string2 now hold simple strings, which means they’re just a sequence of characters stored in these variables. In Python, we can mix single and double quotes like this, which is helpful if you need to use quotes within your string.
Multi-Line String with Trible Quotes
For strings that span multiple lines, Python provides the option to use triple quotes (”’ ”’ or “”” “””). This is handy for writing paragraphs, documentation, or formatted text without adding newline characters manually.
multi_line = ”’This is a multi-line string.”’
multi_line = ”’This is a \nmulti-line string.”’: Here, we’re creating a multi-line string. The triple quotes allow us to hit the Enter key to start a new line directly within the string.
The \n you see automatically at the end of This is a is an escape sequence for “newline,” which Python adds for multi-line strings. It tells Python to continue the text on a new line.
The value of multi_line will look like this when printed:
This is a multi-line string.
Why Use Triple Quotes?
Triple quotes are useful when you want a string that has special formatting, such as line breaks or indents. They’re often used for multi-line comments or docstrings in functions, where you want the string to look just like the block of text you type.
String Methods in Python
Python provides a variety of built-in string methods to perform operations such as searching, replacing, splitting, etc.
Here are some commonly used string methods:
len()
: Returns the length of the string.upper()
: Converts all characters in the string to uppercase.lower()
: Converts all characters in the string to lowercase.replace()
: Replaces a substring with another substring.strip()
: Removes any leading or trailing whitespace.split()
: Splits a string into a list based on a specified delimiter.join()
: Joins elements of a list into a single string.
Python String Indexing and Slicing
In Python, you can access the characters of a string using indices.
text = "Python"
print(text[0]) # Output: P
print(text[-1]) # Output: n
Examples of Python String Methods
- Finding Length of a String
text = "Python"
print(len(text)) # Output: 6
2. Converting to Uppercase
text = "hello world"
print(text.upper()) # Output: HELLO WORLD
3. Replacing Characters
text = "Hello World"
new_text = text.replace("World", "Python")
print(new_text) # Output: Hello Python
4. Splitting Strings
text = "apple,banana,orange"
fruits = text.split(",")
print(fruits) # Output: ['apple', 'banana', 'orange']
Real World Python String Tasks
Now lets see Python in action by solving sole real-world problems. By trying to solve real world problems through coding is the best way to understand and learn programming. The more task or problems you solve through coding, the better programmer you can become. Here are 20 real-world tasks that require string manipulation in Python. These tasks will help you understand how to apply string operations to solve everyday problems.
Task 1: Replacing Characters Without using the replace()
Write a program that replaces every occurrence of one character with another, without using the replace()
method.
codetext = "Hello World"
result = ''
for char in text:
if char == 'e':
result += '6'
elif char == 'l':
result += '9'
else:
result += char
print(result) # Output: H699o Wor9d
Task 2: Extract and Format Telephone Number using Python
Given a string containing a 10-digit phone number, extract the area code, exchange, and number. Then format the output as a phone number.
codenumber = "1234567890"
area_code = number[:3]
exchange = number[3:6]
line_number = number[6:]
print(f"Area: {area_code}\nExchange: {exchange}\nNumber: {line_number}")
print(f"Formatted: ({area_code}){exchange}-{line_number}")
# Output:
# Area: 123
# Exchange: 456
# Number: 7890
# Formatted: (123)456-7890
Task 3: Extract Information from a String using Python
Write a program to extract various details from a string like its length, first and last character, and position of a specific letter.
codetext = "To be or not to be!"
print(f"Length: {len(text)}")
print(f"First character: {text[0]}")
print(f"Last character: {text[-1]}")
print(f"Position of 'r': {text.find('r')}")
Task 4: Remove Spaces from a String using Python
Convert a sentence to remove spaces between words.
codetext = "To be or not to be!"
result = text.replace(" ", "")
print(result) # Output: ToBeOrNotToBe!
Task 5: Convert 2-Digit Year to 4-Digit Year using Python
Convert a 2-digit year format into a 4-digit year format.
codedate = "06/30/98"
date = date[:6] + "19" + date[6:]
print(date) # Output: 06/30/1998
Task 6: Format Name
Format a name into last-name-first format.
codename = "Abdul Jabbar Memon"
parts = name.split()
formatted_name = f"{parts[2]}, {parts[1]} {parts[0][0]}."
print(formatted_name) # Output: Memon, Jabbar A.
Task 7: Convert Number to Words
Write a program that converts a 3-digit number to words.
codenum = 123
words = {
100: "One Hundred",
20: "Twenty",
3: "Three"
}
print(f"{words[100]} {words[20]} {words[3]}")
# Output: One Hundred Twenty Three
Task 8: Date Conversion to Month Format
Convert a date string from “MM/DD/YY” format to “Month DD, YYYY” format.
codedate = "06/30/98"
month_names = {
"06": "June"
}
new_date = f"{month_names[date[:2]]} – {date[3:5]} – 19{date[6:]}"
print(new_date) # Output: June – 30 – 1998
Additional Real-World Python String Tasks
Now its your turn to solve the solve some these questions. solve them and leave the output in the comments below.
- Write a Python function to find all occurrences of a specific substring within a given string.
- Implement a function that capitalizes the first letter of each word in a given string.
- Develop a program to check if a given string is a palindrome.
- Create a function that identifies and returns the most frequent character in a given string.
- Write a Python function to determine if a given string contains only digits.
- Implement a function to count the number of words in a provided sentence.
- Reverse a given string without using built-in methods.
- Write a function to sort the words in a string alphabetically.
- Develop a function that validates if an email format in a given string is correct.
- Create a Python program to remove all punctuation from a given string.
- Write a function to check if two given strings are anagrams.
- Develop a program to find the longest word in a given sentence.
Conclusion:
String manipulation is an essential skill in Python programming. Whether you’re processing user input, generating reports, or cleaning up data, mastering Python’s string methods will give you the tools you need to tackle these tasks efficiently.