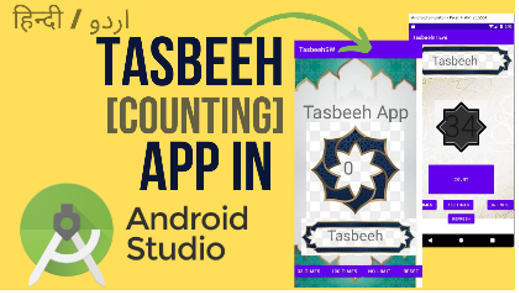
A Tasbeeh Counter App helps users keep track of their prayers, making it easy to reach specific counting goals, like 33 or 100, or to count without any limit. In this tutorial, we’ll build a simple Tasbeeh Counter App in Android Studio using Java, with five buttons:
- Count Button: Increments the tasbeeh count.
- Limit to 33 Button: Sets the counter to stop at 33.
- Limit to 100 Button: Sets the counter to stop at 100.
- No Limit Button: Removes any limit from the counter.
- Reset Button: Resets the counter to zero.
Let’s get started!
Step 1: Set up the Android Studio Project
- Open Android Studio and create a new project.
- Choose Empty Activity and name your project “TasbeehCounterApp”.
- Select Java as the language and proceed to set up the project.
Step 2: Design the XML Layout (activity_main.xml)
We’ll start by creating the layout for the app in activity_main.xml
. This layout will have a TextView
to display the counter, and five buttons for counting, limiting, and resetting the tasbeeh.
Here’s the XML code:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:padding="20dp"
tools:context=".MainActivity">
<!-- Counter Display -->
<TextView
android:id="@+id/tvCounter"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:text="0"
android:textSize="60sp"
android:textColor="#000000"
android:layout_marginBottom="30dp" />
<!-- Count Button -->
<Button
android:id="@+id/btnCount"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Count"
android:textSize="20sp"
android:backgroundTint="#2196F3" />
<!-- Limit to 33 Button -->
<Button
android:id="@+id/btnLimit33"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Limit to 33"
android:textSize="20sp"
android:layout_marginTop="10dp"
android:backgroundTint="#4CAF50" />
<!-- Limit to 100 Button -->
<Button
android:id="@+id/btnLimit100"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Limit to 100"
android:textSize="20sp"
android:layout_marginTop="10dp"
android:backgroundTint="#FF9800" />
<!-- No Limit Button -->
<Button
android:id="@+id/btnNoLimit"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="No Limit"
android:textSize="20sp"
android:layout_marginTop="10dp"
android:backgroundTint="#9C27B0" />
<!-- Reset Button -->
<Button
android:id="@+id/btnReset"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Reset"
android:textSize="20sp"
android:layout_marginTop="10dp"
android:backgroundTint="#F44336" />
</LinearLayout>
Explanation
- TextView (tvCounter): Displays the current tasbeeh count, initialized to “0”.
- Button (btnCount): Increments the counter each time it’s clicked.
- Button (btnLimit33): Sets a limit of 33 on the counter.
- Button (btnLimit100): Sets a limit of 100 on the counter.
- Button (btnNoLimit): Removes any limit, allowing unlimited counting.
- Button (btnReset): Resets the counter back to zero.
Step 3: Add the Logic in Java (MainActivity.java)
Now, let’s add functionality to the buttons in MainActivity.java
.
package com.example.tasbeehcounterapp;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
public class MainActivity extends AppCompatActivity {
private int count = 0;
private int limit = Integer.MAX_VALUE; // Default to no limit
private TextView tvCounter;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Find views by ID
tvCounter = findViewById(R.id.tvCounter);
Button btnCount = findViewById(R.id.btnCount);
Button btnLimit33 = findViewById(R.id.btnLimit33);
Button btnLimit100 = findViewById(R.id.btnLimit100);
Button btnNoLimit = findViewById(R.id.btnNoLimit);
Button btnReset = findViewById(R.id.btnReset);
// Set button listeners
btnCount.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if (count < limit) {
count++;
tvCounter.setText(String.valueOf(count));
}
}
});
btnLimit33.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
limit = 33;
}
});
btnLimit100.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
limit = 100;
}
});
btnNoLimit.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
limit = Integer.MAX_VALUE;
}
});
btnReset.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
count = 0;
tvCounter.setText(String.valueOf(count));
}
});
}
}
Explanation
- Global Variables:
count
: Keeps track of the current tasbeeh count.limit
: Sets a maximum count limit. By default, it’s set toInteger.MAX_VALUE
, meaning no limit.
- onCreate Method:
- This method initializes the views and sets up click listeners for each button.
- btnCount (Increment Counter):
- Checks if the current
count
is below thelimit
. If yes, it increments thecount
and updatestvCounter
to show the new value.
- Checks if the current
- btnLimit33:
- Sets the counter limit to 33 when this button is clicked.
- btnLimit100:
- Sets the counter limit to 100 when this button is clicked.
- btnNoLimit:
- Removes any limit by setting
limit
toInteger.MAX_VALUE
.
- Removes any limit by setting
- btnReset:
- Resets
count
to zero and updates thetvCounter
to reflect the reset.
- Resets
Final Output
Here’s how the app layout should look:
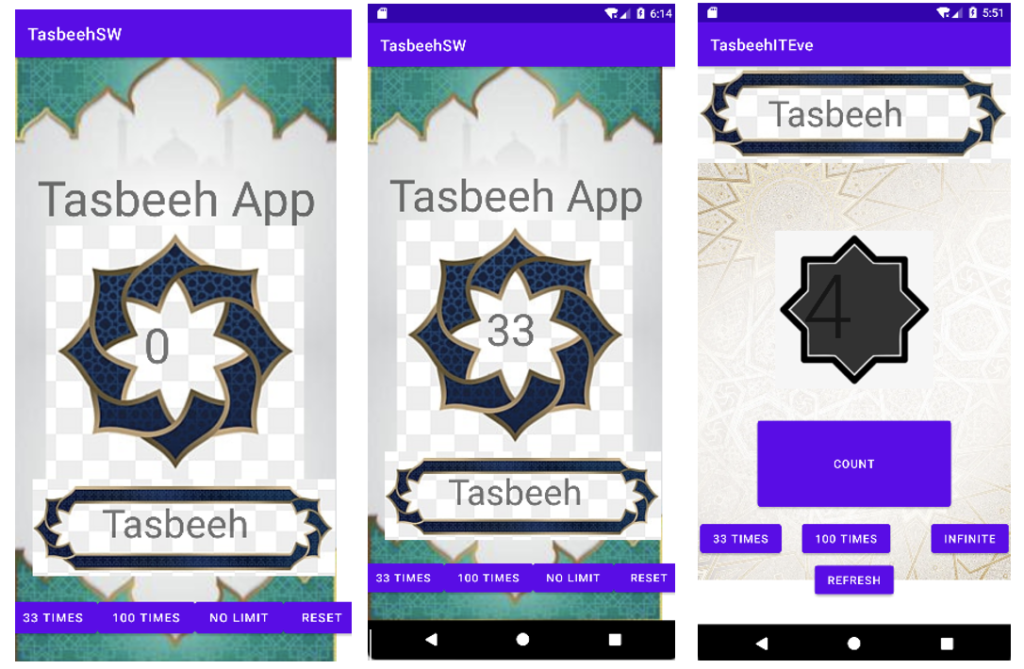
Summary
This app is a simple, effective tool for counting tasbeeh. You can increment the count, set limits (33, 100, or no limit), and reset the count. The app’s functionality can be expanded with more features or additional styling, but this tutorial provides a basic foundation for building a Tasbeeh Counter App in Android Studio using Java.