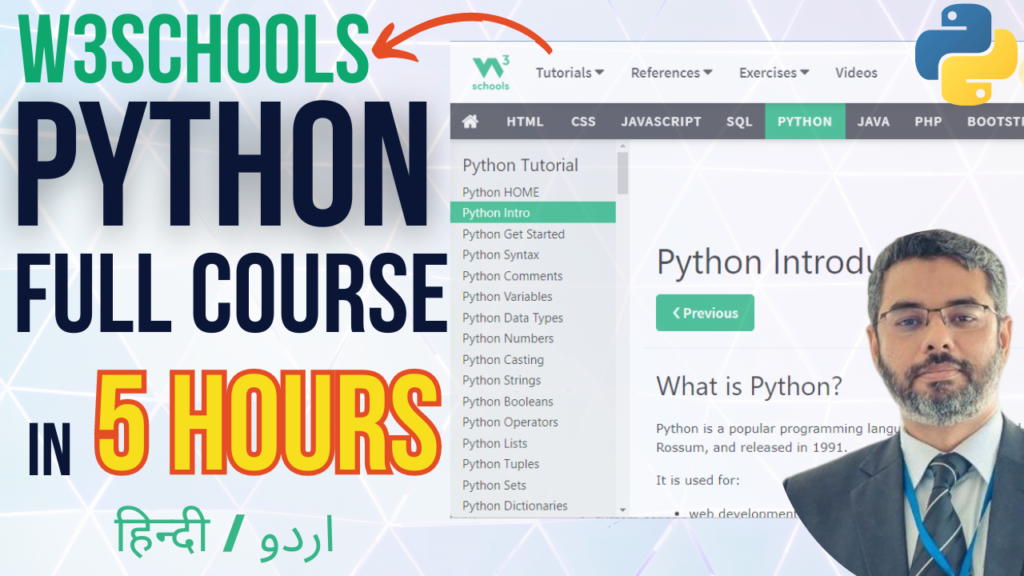
Python Full Coure, Zeeshan Academy
I. Introduction
In Python, strings are one of the most fundamental data types, and they play a crucial role in virtually every Python program. A string is a sequence of characters, and it can be as short as a single character or as long as you need. Python provides a rich set of built-in string functions that allow you to manipulate, analyze, and modify strings easily.
Strings are immutable in Python, meaning once a string is created, it cannot be changed. Instead, most string operations create new strings with the desired modifications. Understanding and mastering string functions is essential for effective text processing, data manipulation, and input validation in Python programming.
In this blog post, we will explore various Python string functions, how to use them effectively, and examples to demonstrate their functionalities. By the end of this guide, you’ll have a solid understanding of Python string manipulation and be able to apply these concepts to solve real-world programming challenges.
A. Overview of Python’s built-in string functions
Python’s standard library provides a wide range of built-in functions specifically designed to work with strings. Some of the most commonly used string functions include:
- String Concatenation: Combining two or more strings together to create a new string.
- String Slicing: Extracting substrings or accessing individual characters from a string.
- String Length: Finding the number of characters in a string using the ‘len()’ function.
- String Case Conversion: Changing the case of a string (uppercase, lowercase, etc.).
- Searching and Replacing: Searching for substrings and replacing them with desired values.
- String Splitting and Joining: Splitting a string into a list of substrings and joining them back together.
- String Stripping: Removing leading and trailing whitespace or specific characters from a string.
- String Formatting: Creating formatted strings using placeholders and replacement fields.
- Checking String Properties: Validating strings for alphanumeric characters, digits, lowercase, etc.
- Miscellaneous String Functions: Checking if a string starts or ends with a particular substring, etc.
In the upcoming sections, we’ll delve into each of these string functions, providing detailed explanations and practical examples to illustrate their usage.
Stay tuned for the next section, where we’ll explore common string operations, including string concatenation, slicing, and finding string length. Let’s dive in!
Sure! Let’s go into detail for Section 1 and Section 2, providing suitable examples for each Python string operation:
II. Common String Operations
A. String Concatenation
String concatenation is the process of combining two or more strings into a single string.
1. Using the ‘+’ operator:
You can concatenate strings using the ‘+’ operator.
# Example: string1 = "Hello, " string2 = "Python!" result = string1 + string2 print(result) # Output: "Hello, Python!"
2. Using the ‘join()’ method:
The ‘join()’ method is used to concatenate elements of an iterable, such as a list, using a separator string.
# Example: words = ["Welcome", "to", "Python", "programming"] separator = " " result = separator.join(words) print(result) # Output: "Welcome to Python programming"
B. String Slicing
String slicing allows you to extract substrings or access individual characters from a string.
1. Extracting substrings:
You can extract a portion of a string using slicing. The syntax for slicing is string[start_index:stop_index]
, where the start_index
is inclusive and the stop_index
is exclusive.
# Example: sentence = "Python is amazing" substring = sentence[0:6] # Extracts characters from index 0 to 5 (inclusive). print(substring) # Output: "Python"
2. Accessing individual characters:
You can access individual characters from a string using indexing.
# Example: text = "Hello" first_char = text[0] last_char = text[-1] # Negative index counts from the end of the string. print(first_char) # Output: "H" print(last_char) # Output: "o"
C. String Length
String length refers to the number of characters in a string.
1. Using ‘len()’ function:
The ‘len()’ function returns the length of the string.
# Example: message = "Hello, world!" length = len(message) print(length) # Output: 13
2. Handling empty strings:
Empty strings have a length of 0.
# Example: empty_string = "" length = len(empty_string) print(length) # Output: 0
These are some of the common string operations in Python. They allow you to manipulate and extract information from strings efficiently, which is crucial for many programming tasks.
III. Common String Operations
A. String Concatenation
String concatenation is the process of combining two or more strings to create a new string. It allows you to join different pieces of text or data into a single string.
1. Using the ‘+’ operator:
You can use the ‘+’ operator to concatenate strings. When the ‘+’ operator is used between two strings, it merges them into one.
# Example: string1 = "Hello, " string2 = "Python!" result = string1 + string2 print(result) # Output: "Hello, Python!"
2. Using the ‘join()’ method:
The ‘join()’ method is an alternative approach to concatenate strings, especially when you have a list of strings that you want to join together with a separator.
# Example: words = ["Welcome", "to", "Python", "programming"] separator = " " result = separator.join(words) print(result) # Output: "Welcome to Python programming"
In the first example, we used the ‘+’ operator to concatenate the two strings, while in the second example, we joined the elements of the ‘words’ list with a space as the separator.
String concatenation is a basic yet essential operation when building text-based applications or composing dynamic output. Understanding both methods will help you efficiently combine strings in your Python programs.
Now, let’s move on to the next subsection, where we’ll explore string slicing, which allows you to extract substrings and access individual characters from a string. Stay with us!
Of course! Let’s continue with the detailed descriptions for the remaining sections of your blog post on Python String Functions:
B. String Slicing
String slicing is a powerful technique that allows you to extract substrings or access individual characters from a string. This operation is particularly useful when you need to work with specific parts of a string.
1. Extracting substrings:
To extract a substring from a given string, you can use slicing. The syntax for slicing is string[start_index:stop_index]
, where the start_index
is inclusive, and the stop_index
is exclusive.
# Example: original_string = "Python is versatile and powerful" substring = original_string[7:15] # Extracts characters from index 7 to 14 (inclusive). print(substring) # Output: "is versat"
2. Accessing individual characters:
You can access individual characters from a string using indexing. Positive indices count from the beginning of the string (starting at 0), while negative indices count from the end of the string.
# Example: text = "Hello" first_char = text[0] last_char = text[-1] # Negative index counts from the end of the string. print(first_char) # Output: "H" print(last_char) # Output: "o"
C. String Length
The length of a string, i.e., the number of characters it contains, is a fundamental property that is often needed in programming.
1. Using ‘len()’ function:
The built-in len()
function returns the length of a string.
# Example: message = "Hello, world!" length = len(message) print(length) # Output: 13
2. Handling empty strings:
An empty string has a length of 0.
# Example: empty_string = "" length = len(empty_string) print(length) # Output: 0
Understanding string slicing and finding string length are key skills for manipulating and extracting information from strings. These operations form the foundation for more advanced string manipulations and transformations that you’ll explore in the upcoming sections.
In the next section, we’ll dive into string case conversion, where you’ll learn how to change the case of strings in Python to suit your needs. Stay tuned!
IV. String Case Conversion
String case conversion involves changing the letter case of a string. Python provides several methods to convert strings to uppercase, lowercase, and even swap the case.
A. Converting to Uppercase
1. ‘upper()’ method:
The ‘upper()’ method converts all characters in a string to uppercase.
# Example: message = "hello, world!" uppercase_message = message.upper() print(uppercase_message) # Output: "HELLO, WORLD!"
2. ‘capitalize()’ method:
The ‘capitalize()’ method capitalizes the first character of the string, making all other characters lowercase.
# Example: text = "hello, world!" capitalized_text = text.capitalize() print(capitalized_text) # Output: "Hello, world!"
B. Converting to Lowercase
1. ‘lower()’ method:
The ‘lower()’ method converts all characters in a string to lowercase.
# Example: message = "Hello, World!" lowercase_message = message.lower() print(lowercase_message) # Output: "hello, world!"
2. ‘title()’ method:
The ‘title()’ method converts the first character of each word in the string to uppercase, while making all other characters lowercase. It is useful for transforming sentence-like strings.
# Example: sentence = "python programming is fun" title_case_sentence = sentence.title() print(title_case_sentence) # Output: "Python Programming Is Fun"
C. Swapping Case
1. ‘swapcase()’ method:
The ‘swapcase()’ method swaps the case of each character in the string, converting uppercase characters to lowercase and vice versa.
# Example: text = "hElLo, WoRlD!" swapped_text = text.swapcase() print(swapped_text) # Output: "HeLlO, wOrLd!"
String case conversion is often used to standardize input data or display strings in a consistent format. Depending on your application’s requirements, you can use these methods to convert strings to the desired case format.
Next, in Section IV, we’ll cover searching and replacing substrings in Python strings. These operations are useful when you need to find specific patterns or modify parts of a string. Stay tuned!
V. Searching and Replacing
Searching and replacing substrings within a string is a common task in text processing. Python provides several built-in string methods to help you efficiently search for substrings and replace them with desired values.
A. Finding Substrings
1. ‘find()’ and ‘rfind()’ methods:
The ‘find()’ method is used to find the first occurrence of a substring in the given string. It returns the index of the first character of the substring if found, or -1 if the substring is not present.
# Example: text = "Python is easy to learn and fun to use" search_string = "easy" index = text.find(search_string) print(index) # Output: 10
The ‘rfind()’ method works similarly, but it searches the string from right to left and returns the last occurrence of the substring.
# Example: text = "Python is easy to learn and fun to use" search_string = "to" index = text.rfind(search_string) print(index) # Output: 23
2. ‘index()’ and ‘rindex()’ methods:
The ‘index()’ method is similar to ‘find()’, but it raises a ValueError if the substring is not found.
# Example: text = "Python is easy to learn and fun to use" search_string = "use" index = text.index(search_string) print(index) # Output: 35
The ‘rindex()’ method is similar to ‘rfind()’, but it raises a ValueError if the substring is not found.
# Example: text = "Python is easy to learn and fun to use" search_string = "easy" index = text.rindex(search_string) print(index) # Output: 10
B. Counting Occurrences
‘count()’ method:
The ‘count()’ method is used to count the number of occurrences of a substring in the given string.
# Example: text = "Python is easy to learn and fun to use" search_string = "o" occurrences = text.count(search_string) print(occurrences) # Output: 3
C. Replacing Substrings
‘replace()’ method:
The ‘replace()’ method is used to replace all occurrences of a substring with a new value.
# Example: text = "Python is easy to learn and fun to use" search_string = "easy" replacement = "simple" new_text = text.replace(search_string, replacement) print(new_text) # Output: "Python is simple to learn and fun to use"
Searching and replacing substrings in Python strings allows you to modify text and data effectively. These methods are valuable for data cleaning, data parsing, and data manipulation tasks.
Next, in Section V, we’ll explore string splitting and joining techniques, which are essential for breaking strings into parts and combining them back together. Keep reading!
VI. String Splitting and Joining
String splitting and joining are important operations that allow you to break a string into parts or combine multiple strings into one. These operations are widely used in data processing and text manipulation tasks.
A. Splitting a String
1. ‘split()’ method:
The ‘split()’ method is used to split a string into a list of substrings based on a specified separator. By default, the separator is any whitespace (spaces, tabs, or newlines).
# Example: text = "Python is fun to learn" words = text.split() print(words) # Output: ['Python', 'is', 'fun', 'to', 'learn']
You can also specify a custom separator by passing it as an argument to the ‘split()’ method.
# Example: text = "apple,banana,orange" fruits = text.split(',') print(fruits) # Output: ['apple', 'banana', 'orange']
2. ‘splitlines()’ method:
The ‘splitlines()’ method splits a string into a list of substrings based on the newline character (‘\n’).
# Example: text = "First line\nSecond line\nThird line" lines = text.splitlines() print(lines) # Output: ['First line', 'Second line', 'Third line']
B. Joining Strings
‘join()’ method:
The ‘join()’ method is used to concatenate a sequence of strings using a specified separator. It takes an iterable (e.g., a list or tuple) as an argument and returns a single string formed by joining the elements of the iterable with the separator.
# Example: words = ['Python', 'is', 'fun', 'to', 'learn'] separator = ' ' text = separator.join(words) print(text) # Output: "Python is fun to learn"
You can also join strings without any separator by passing an empty string as the argument to the ‘join()’ method.
# Example: words = ['apple', 'banana', 'orange'] text = ''.join(words) print(text) # Output: "applebananaorange"
String splitting and joining are essential techniques for processing textual data, parsing information, and reassembling strings in different formats. These operations play a crucial role in various data manipulation scenarios.
In the next section, we’ll explore string stripping, which allows you to remove unwanted leading and trailing characters from a string. Stay tuned for more string manipulation goodness!
VII. String Stripping
String stripping is the process of removing leading and trailing characters, such as whitespaces or specific characters, from a string. This is useful for cleaning up user inputs or processing data with unnecessary padding.
A. Removing Whitespaces
1. ‘strip()’, ‘lstrip()’, and ‘rstrip()’ methods:
The ‘strip()’ method is used to remove leading and trailing whitespaces from a string.
# Example: text = " Python is fun " stripped_text = text.strip() print(stripped_text) # Output: "Python is fun"
The ‘lstrip()’ method removes leading whitespaces only, and the ‘rstrip()’ method removes trailing whitespaces only.
# Example: text = " Python is fun " left_stripped_text = text.lstrip() right_stripped_text = text.rstrip() print(left_stripped_text) # Output: "Python is fun " print(right_stripped_text) # Output: " Python is fun"
B. Removing Specific Characters
‘strip()’ with argument:
The ‘strip()’ method can also remove specific characters from the beginning and end of a string by passing a string containing the characters to remove.
# Example: text = "-----Python is fun!!!----" stripped_text = text.strip('-!') print(stripped_text) # Output: "Python is fun"
String stripping is essential when dealing with user inputs, reading data from files, or processing text from external sources. It ensures that strings are cleaned and ready for further processing or analysis.
In the next section, we’ll explore different string formatting techniques that allow you to create well-organized and visually appealing output. Stay tuned to learn more about Python string formatting!
VIII. String Formatting
String formatting is a powerful technique used to create well-organized and visually appealing output. Python provides various methods for formatting strings, allowing you to include variables, numbers, and other data types within your text.
A. Using f-strings
F-strings, also known as formatted string literals, are a popular and concise way to format strings in Python. They allow you to embed expressions inside string literals by placing them within curly braces {}. When the f-string is evaluated, the expressions are replaced with their values.
# Example: name = "Alice" age = 30 message = f"Hello, my name is {name} and I am {age} years old." print(message) # Output: "Hello, my name is Alice and I am 30 years old."
You can also perform computations and use formatting options inside the curly braces.
# Example: price = 19.99 discount = 0.2 final_price = f"The final price is ${price * (1 - discount):.2f}." print(final_price) # Output: "The final price is $15.99."
B. Using the ‘format()’ method
The ‘format()’ method is an older method for string formatting, which offers more flexibility in certain situations.
# Example: name = "Bob" age = 25 message = "Hello, my name is {} and I am {} years old.".format(name, age) print(message) # Output: "Hello, my name is Bob and I am 25 years old."
You can also use positional and keyword arguments for better control over the order of replacements.
# Example: product = "phone" price = 499.99 message = "The {} costs ${:.2f}.".format(product, price) print(message) # Output: "The phone costs $499.99."
String formatting allows you to create dynamic and informative output that adapts to the data you have. Choose the method that best suits your needs and enhances the readability of your code.
In the next section, we’ll explore different checks you can perform on strings to determine specific properties like whether a string is numeric, alphabetic, or contains only whitespace. Stay tuned for more insights into Python string checks!
IX. Checking String Properties
Python provides several methods to check various properties of a string. These checks are useful when you need to validate or categorize strings based on certain criteria.
A. Checking if a String is Numeric
‘isnumeric()’ method:
The ‘isnumeric()’ method checks whether all characters in a string are numeric (i.e., digits).
# Example: number = "12345" result = number.isnumeric() print(result) # Output: True text = "Hello" result = text.isnumeric() print(result) # Output: False
B. Checking if a String is Alphabetic
‘isalpha()’ method:
The ‘isalpha()’ method checks whether all characters in a string are alphabetic (i.e., letters).
# Example: word = "Python" result = word.isalpha() print(result) # Output: True text = "Hello123" result = text.isalpha() print(result) # Output: False
C. Checking if a String is Numeric with Exceptions
‘isdigit()’ method:
The ‘isdigit()’ method checks whether all characters in a string are digits. However, it will return False if the string contains any non-digit characters, including whitespace.
# Example: number = "12345" result = number.isdigit() print(result) # Output: True text = "12 345" result = text.isdigit() print(result) # Output: False
D. Checking if a String is in Lowercase or Uppercase
‘islower()’ and ‘isupper()’ methods:
The ‘islower()’ method checks if all characters in a string are lowercase, while ‘isupper()’ checks if all characters are uppercase.
# Example: text1 = "hello" result1 = text1.islower() print(result1) # Output: True text2 = "Hello" result2 = text2.isupper() print(result2) # Output: False
E. Checking if a String Contains Only Whitespace
‘isspace()’ method:
The ‘isspace()’ method checks if a string contains only whitespace characters (spaces, tabs, or newlines).
# Example: whitespace_text = " \t \n " result = whitespace_text.isspace() print(result) # Output: True text = "Hello, world!" result = text.isspace() print(result) # Output: False
String property checks are useful for data validation and filtering tasks. They help you ensure that strings meet specific criteria before further processing.
In the next section, we’ll explore miscellaneous string functions that can come in handy in various situations. Stay tuned to uncover more Python string magic!
X. Miscellaneous String Functions
Python provides a variety of miscellaneous string functions that can be useful in different scenarios. These functions offer additional capabilities for tasks like checking if a string starts or ends with a particular substring, identifying the type of characters in a string, and more.
A. ‘startswith()’ and ‘endswith()’ methods
‘startswith()’ method:
The ‘startswith()’ method checks if a string starts with a specified prefix.
# Example: text = "Hello, world!" result = text.startswith("Hello") print(result) # Output: True result = text.startswith("world") print(result) # Output: False
‘endswith()’ method:
The ‘endswith()’ method checks if a string ends with a specified suffix.
# Example: text = "Hello, world!" result = text.endswith("world!") print(result) # Output: True result = text.endswith("Hello") print(result) # Output: False
B. Character Type Checks
‘isalnum()’, ‘isalpha()’, ‘isdigit()’, ‘islower()’, ‘isupper()’ methods
These methods allow you to determine the type of characters present in a string.
# Example: text1 = "Hello123" result1 = text1.isalnum() # Checks if all characters are alphanumeric. print(result1) # Output: True text2 = "Hello" result2 = text2.isalpha() # Checks if all characters are alphabetic. print(result2) # Output: True text3 = "123" result3 = text3.isdigit() # Checks if all characters are digits. print(result3) # Output: True text4 = "hello" result4 = text4.islower() # Checks if all characters are lowercase. print(result4) # Output: True text5 = "WORLD" result5 = text5.isupper() # Checks if all characters are uppercase. print(result5) # Output: True
C. ‘isspace()’ method
The ‘isspace()’ method we covered earlier also falls under this category. It checks if a string contains only whitespace characters.
# Example: text = " \t \n " result = text.isspace() print(result) # Output: True text = "Hello, world!" result = text.isspace() print(result) # Output: False
These miscellaneous string functions provide additional tools for working with strings and can be handy in various situations. Understanding and utilizing them can enhance the flexibility and robustness of your Python programs.
In the next section, we’ll conclude the blog post with a summary and recap of the essential Python string functions covered. Stay tuned for the final part of this comprehensive guide!
X. Conclusion
In this comprehensive guide, we explored various essential Python string functions that allow you to manipulate, analyze, and transform strings with ease. We covered a wide range of operations, from basic string concatenation and slicing to more advanced tasks like string case conversion, searching, replacing, splitting, and joining.
Here’s a quick recap of the main Python string functions covered in this blog post:
- Common String Operations
- String Concatenation using the ‘+’ operator and ‘join()’ method.
- String Slicing to extract substrings and access individual characters.
- Finding String Length using the ‘len()’ function.
2. String Case Conversion
- Converting to Uppercase using the ‘upper()’ and ‘capitalize()’ methods.
- Converting to Lowercase using the ‘lower()’ and ‘title()’ methods.
- Swapping Case using the ‘swapcase()’ method.
3. Searching and Replacing
- Finding Substrings using ‘find()’, ‘rfind()’, ‘index()’, and ‘rindex()’ methods.
- Counting Occurrences with the ‘count()’ method.
- Replacing Substrings using the ‘replace()’ method.
4. String Splitting and Joining
- Splitting a String using the ‘split()’ and ‘splitlines()’ methods.
- Joining Strings using the ‘join()’ method.
5. String Stripping
- Removing Whitespaces using ‘strip()’, ‘lstrip()’, and ‘rstrip()’ methods.
- Removing Specific Characters with ‘strip()’ and an argument.
6. String Formatting
- Using f-strings for formatted string literals.
- Using the ‘format()’ method for flexible string formatting.
7. Checking String Properties
- Checking if a String is Numeric with ‘isnumeric()’ method.
- Checking if a String is Alphabetic using ‘isalpha()’ method.
- Checking if a String is Numeric with Exceptions using ‘isdigit()’ method.
- Checking if a String is in Lowercase or Uppercase using ‘islower()’ and ‘isupper()’ methods.
- Checking if a String Contains Only Whitespace using ‘isspace()’ method.
8. Miscellaneous String Functions
- ‘startswith()’ and ‘endswith()’ methods for checking prefixes and suffixes.
- Character Type Checks: ‘isalnum()’, ‘isalpha()’, ‘isdigit()’, ‘islower()’, ‘isupper()’ methods.
- ‘isspace()’ method to check if a string contains only whitespace.
By mastering these string functions, you’ll be well-equipped to handle various text processing and data manipulation tasks in Python. Remember that strings are a fundamental aspect of programming, and a strong understanding of string manipulation can greatly enhance your ability to create powerful and efficient Python applications.
Happy coding with Python!