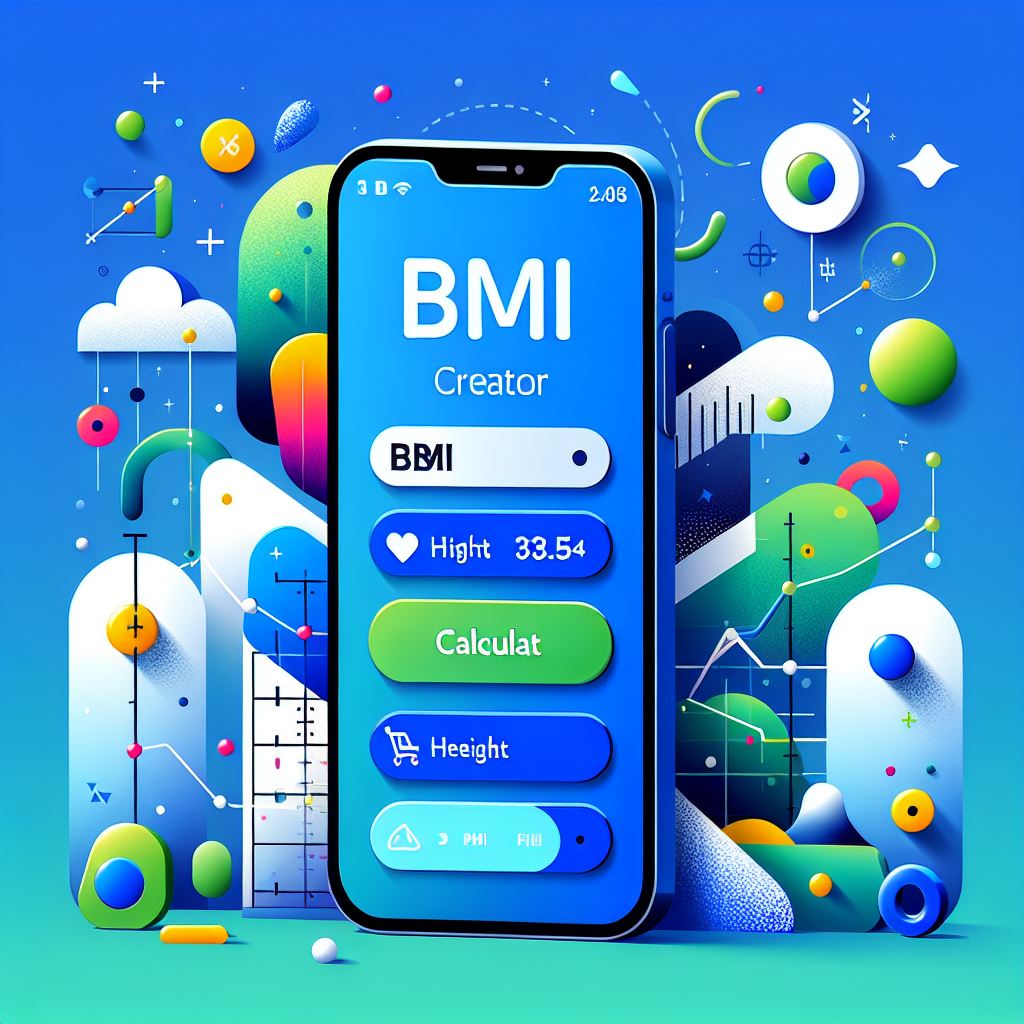
1. Introduction
The BMI Calculator app is a mobile application developed using the Flutter framework that allows users to calculate their Body Mass Index (BMI) based on their input of height and weight. The app aims to provide a quick and easy way for users to assess their body’s health status and fitness level.
2. User Requirements
2.1. User Inputs
The app shall provide a user-friendly interface where users can input their height and weight.
2.2. Metric and Imperial Units
The app shall support both metric (centimeters/kilograms) and imperial (inches/pounds) units for height and weight input.
2.3. Validations
The app shall validate user inputs to ensure they are within reasonable ranges (e.g., height > 0, weight > 0).
2.4. Calculate BMI
Upon user input of height and weight, the app shall calculate the BMI using the following formula:
BMI = weight (kg) / (height (m) * height (m))
For imperial units:
BMI = (weight (lbs) / (height (in) * height (in))) * 703
2.5. BMI Classification
The app shall classify the calculated BMI into categories such as underweight, normal weight, overweight, and obese, according to standard BMI ranges.
2.6. Display Results
The app shall display the calculated BMI value and its corresponding classification to the user.
3. System Requirements
3.1. Platform Compatibility
The BMI Calculator app shall be developed for both Android and iOS platforms, ensuring compatibility with a wide range of devices.
3.2. User Interface
The app shall have an intuitive and visually appealing user interface that makes it easy for users to input their data and view the results.
4. Development Requirements
4.1. Programming Language
The app shall be developed using the Flutter framework with Dart as the primary programming language.
4.2. BMI Calculation
The app shall implement the necessary logic for accurately calculating the BMI based on user inputs and the chosen unit system.
4.3. BMI Classification
The app shall implement the logic to classify the BMI result into appropriate categories based on standard BMI ranges.
4.4. Unit Conversion
The app shall include functionality to convert height and weight between metric and imperial units.
4.5. Testing
The app shall undergo rigorous testing to ensure accurate calculations, proper validations, and a smooth user experience.
5. User Documentation
The app shall provide clear and concise user documentation, including instructions on how to use the BMI Calculator and interpret the results.
6. Future Enhancements
The app may include additional features in the future, such as a history of previous BMI calculations, personalized health tips, and integration with health tracking platforms.
This Simple Requirements Specification outlines the basic functionalities and features of the BMI Calculator app. Additional details and design considerations can be added as the project progresses.
BMI Calculator App – UI Design and Requirements
1. App Theme and Style
The app should have a clean and modern design, with a focus on user-friendliness and readability. Consider using a light color scheme with contrasting colors to make the interface visually appealing.
2. Home Screen
Upon opening the app, users should see a simple and welcoming home screen with the following elements:
- App logo and title at the top of the screen.
- Two input fields for height and weight, along with dropdowns to select the unit system (metric or imperial).
- A “Calculate” button below the input fields.
3. Input Validation
Ensure that the app performs input validation for height and weight fields. If the user enters invalid values (e.g., negative height/weight), display an error message to prompt them to enter valid data.
4. BMI Calculation and Classification
After the user clicks the “Calculate” button, the app should display the calculated BMI value and its corresponding classification on a new screen. Show the BMI value prominently, and use a color-coded label (e.g., green for normal weight, red for overweight) to represent the BMI category.
5. Results Screen
The results screen should include the following elements:
- The calculated BMI value.
- A text label indicating the BMI classification (e.g., “Normal Weight,” “Underweight,” “Overweight,” “Obese”).
- A brief description of what the BMI value means and its implications for the user’s health.
6. Unit Conversion
If the user selects the imperial unit system, the app should convert the input values to metric units before performing the BMI calculation. Display the converted values on the results screen for clarity.
7. Navigation
Include a clear and intuitive navigation system that allows users to move between the home screen and results screen effortlessly.
8. Responsive Design
Ensure that the app’s UI is responsive and adapts to different screen sizes and orientations for both Android and iOS devices.
9. User Settings
Consider adding a settings page where users can set their preferred unit system (metric or imperial) and customize other app-related preferences.
10. User Documentation and Help
Include a help or information icon that users can tap to access a user guide or explanation of how to use the app effectively.
11. Accessibility
Ensure that the app meets accessibility standards, such as providing support for screen readers and making the interface easily navigable for users with disabilities.
12. App Icon
Design an attractive and relevant app icon that represents the BMI Calculator’s purpose.
13. Future Enhancements
Keep the app design flexible to accommodate potential future enhancements, such as integrating with health tracking devices, providing personalized health tips, and storing BMI history for users.
By implementing these UI design and requirements, the BMI Calculator app will offer users a seamless and user-friendly experience while helping them assess their BMI and overall health status.
BMI Calculator App – Splash Screen
Let’s create a splash screen in Flutter with the app logo and title. For this example, assume that you have already prepared the app logo image and stored it in the assets folder. Here’s the code to create the splash screen:
- First, add the app logo image to your project. Place the logo image (e.g.,
app_logo.png
) in theassets/images/
folder of your Flutter project. - Update the
pubspec.yaml
file to include the app logo in the asset bundle:
flutter: assets: - assets/images/app_logo.png
- Now, let’s create the Flutter code for the splash screen. In your main.dart file, add the following code:
import 'package:flutter/material.dart'; void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( title: 'BMI Calculator', theme: ThemeData( primarySwatch: Colors.blue, ), home: SplashScreen(), ); } } class SplashScreen extends StatefulWidget { @override _SplashScreenState createState() => _SplashScreenState(); } class _SplashScreenState extends State<SplashScreen> { @override void initState() { super.initState(); // Add a delay to simulate a splash screen duration (e.g., 2 seconds). Future.delayed(Duration(seconds: 2), () { // Navigate to the home screen after the splash screen duration. Navigator.pushReplacement( context, MaterialPageRoute(builder: (context) => HomeScreen()), ); }); } @override Widget build(BuildContext context) { return Scaffold( backgroundColor: Colors.blue, // Customize the background color as needed. body: Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, children: [ Image.asset( 'assets/images/app_logo.png', // Path to the app logo image. width: 150, height: 150, // You can customize the logo size as needed. ), SizedBox(height: 16), Text( 'BMI Calculator', style: TextStyle( color: Colors.white, // Customize the title color as needed. fontSize: 24, fontWeight: FontWeight.bold, ), ), ], ), ), ); } } class HomeScreen extends StatelessWidget { @override Widget build(BuildContext context) { // Add your BMI calculator UI and logic here. return Scaffold( appBar: AppBar( title: Text('BMI Calculator'), ), body: Center( child: Text('Your BMI Calculator UI goes here'), ), ); } }
The code above creates a simple splash screen with the app logo and title using the SplashScreen
widget. We use the initState
method to add a delay of 2 seconds before navigating to the HomeScreen
. This delay simulates the splash screen duration. Once the delay is complete, we replace the splash screen with the HomeScreen
.
You can customize the background color, logo size, title style, and splash screen duration according to your app’s design and requirements.
The Home Screen
Let’s create the home screen code for the BMI Calculator app. In this example, we’ll implement the UI for user input of height, weight, and the BMI calculation. Here’s the code for the home screen:
- First, update the
HomeScreen
widget in themain.dart
file as follows:
class HomeScreen extends StatefulWidget { @override _HomeScreenState createState() => _HomeScreenState(); } class _HomeScreenState extends State<HomeScreen> { // Variables to store user input data. double height = 0; double weight = 0; String selectedUnit = 'Metric'; // Function to calculate the BMI. double calculateBMI() { double bmi; if (selectedUnit == 'Metric') { bmi = weight / ((height / 100) * (height / 100)); } else { bmi = (weight / (height * height)) * 703; } return bmi; } // Function to get the BMI classification. String getBMIClassification(double bmi) { if (bmi < 18.5) { return 'Underweight'; } else if (bmi >= 18.5 && bmi < 24.9) { return 'Normal Weight'; } else if (bmi >= 25 && bmi < 29.9) { return 'Overweight'; } else { return 'Obese'; } } @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text('BMI Calculator'), ), body: Padding( padding: const EdgeInsets.all(16.0), child: Column( mainAxisAlignment: MainAxisAlignment.center, children: [ // Height input TextFormField( keyboardType: TextInputType.number, decoration: InputDecoration( labelText: 'Height', hintText: selectedUnit == 'Metric' ? 'cm' : 'in', ), onChanged: (value) { setState(() { height = double.tryParse(value) ?? 0; }); }, ), SizedBox(height: 16), // Weight input TextFormField( keyboardType: TextInputType.number, decoration: InputDecoration( labelText: 'Weight', hintText: selectedUnit == 'Metric' ? 'kg' : 'lbs', ), onChanged: (value) { setState(() { weight = double.tryParse(value) ?? 0; }); }, ), SizedBox(height: 16), // Unit selection dropdown DropdownButton<String>( value: selectedUnit, onChanged: (newValue) { setState(() { selectedUnit = newValue!; }); }, items: ['Metric', 'Imperial'] .map<DropdownMenuItem<String>>((String value) { return DropdownMenuItem<String>( value: value, child: Text(value), ); }).toList(), ), SizedBox(height: 16), // Calculate BMI button ElevatedButton( onPressed: () { double bmi = calculateBMI(); String classification = getBMIClassification(bmi); // Show the results screen with the calculated BMI. Navigator.push( context, MaterialPageRoute( builder: (context) => ResultsScreen( bmi: bmi, classification: classification, ), ), ); }, child: Text('Calculate BMI'), ), ], ), ), ); } }
In the updated HomeScreen
widget, we have added text input fields for height and weight, along with a dropdown button to select the unit system (metric or imperial). The user’s input is stored in the height
, weight
, and selectedUnit
variables.
The calculateBMI()
function calculates the BMI based on the user’s input and the selected unit system. The getBMIClassification()
function returns the BMI classification based on the calculated BMI value.
When the user clicks the “Calculate BMI” button, the app navigates to the ResultsScreen
, passing the calculated BMI value and classification as arguments.
Now, we need to create the ResultsScreen
widget to display the BMI calculation results.
- Create a new file named
results_screen.dart
in your project and add the following code:
import 'package:flutter/material.dart'; class ResultsScreen extends StatelessWidget { final double bmi; final String classification; ResultsScreen({ required this.bmi, required this.classification, }); @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text('BMI Results'), ), body: Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, children: [ Text( 'Your BMI: ${bmi.toStringAsFixed(1)}', style: TextStyle(fontSize: 24, fontWeight: FontWeight.bold), ), SizedBox(height: 16), Text( 'Classification: $classification', style: TextStyle(fontSize: 18), ), ], ), ), ); } }
In the ResultsScreen
widget, we display the calculated BMI and its classification based on the arguments passed from the HomeScreen
. The BMI value is formatted to display one decimal place using toStringAsFixed(1)
.
That’s it! Now you have the home screen code for the BMI Calculator app, along with the results screen to display the calculated BMI. Remember to import the required packages in both files and run your app to see the BMI Calculator in action.