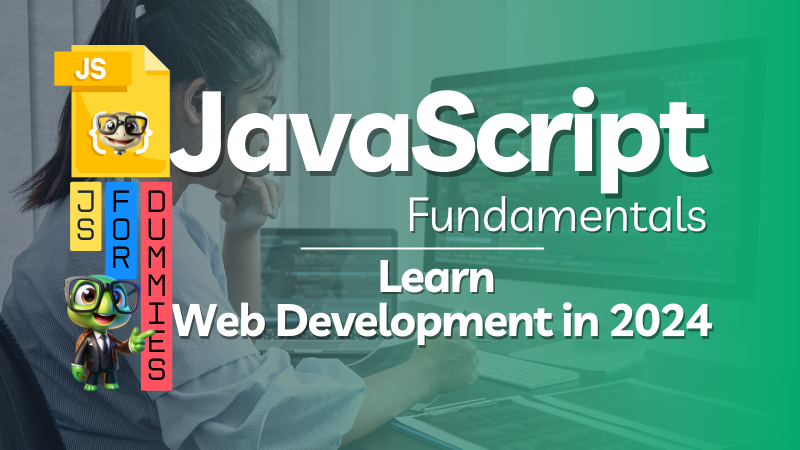
Discover the ultimate roadmap to mastering JavaScript for web development in 2024. Learn step-by-step techniques, essential syntax, practical examples, and FAQs to become a JavaScript expert fast.
Introduction: Why Learn JavaScript in 2024?
JavaScript is the backbone of modern web development. From creating interactive websites to powering frameworks like React, Angular, and Vue.js, JavaScript remains the go-to language for developers. In 2024, its demand is skyrocketing as businesses continue to prioritize user-friendly digital experiences.
This guide outlines the best ways to learn JavaScript for web development, emphasizing practical steps, detailed syntax explanations, and examples to help you become proficient.
Table of Contents
- 1. Getting Started with JavaScript
- 2. Setting Up Your Development Environment
- 3. Learning Basic Syntax and Concepts
- 4. Understanding JavaScript Variables and Data Types
- 5. Mastering Functions in JavaScript
- 6. Diving into Control Flow Statements
- 7. Exploring Objects and Arrays
- 8. Event Handling for Dynamic Web Pages
- 9. Learning Asynchronous JavaScript: Promises and Async/Await
- 10. Building Real-World Projects
- FAQs
- Conclusion
1. Getting Started with JavaScript
JavaScript is a versatile language that runs in the browser and on servers using Node.js. Here’s why JavaScript is essential:
- Cross-Platform: Works on web browsers, mobile apps, and servers.
- Large Community: Access to countless resources and libraries.
- High Demand: Web development heavily relies on JavaScript.
2. Setting Up Your Development Environment
To begin coding, follow these steps:
- Install a Code Editor:
- VS Code: Lightweight, feature-rich, and widely used.
- Example:
- Open Visual Studio Code and install it.
- Install Node.js:
- Node.js allows you to run JavaScript outside the browser.
- Example Command:
node -v npm -v
- Choose a Browser Developer Tool:
- Chrome DevTools or Firefox Developer Tools are excellent options for debugging.
3. Learning Basic Syntax and Concepts
JavaScript Basics
Here’s a quick rundown of the syntax:
- Hello World Example:
console.log("Hello, World!");
- Comments:
- Single-line:
// This is a comment
- Multi-line:
/* This is a multi-line comment */
- Single-line:
- Semicolons: Optional but recommended for clarity.
4. Understanding JavaScript Variables and Data Types
Variables
JavaScript uses var
, let
, and const
for declaring variables.
Example 1: var
var name = "Alice";
console.log(name);
Example 2: let
let age = 25;
console.log(age);
Example 3: const
const PI = 3.14;
console.log(PI);
Data Types
- String
let greeting = "Hello";
- Number
let score = 100;
- Boolean
let isLoggedIn = true;
5. Mastering Functions in JavaScript
Functions are reusable blocks of code.
Syntax
function functionName(parameters) {
// Code to execute
}
Example 1: Simple Function
function sayHello() {
console.log("Hello!");
}
sayHello();
Example 2: Function with Parameters
function add(a, b) {
return a + b;
}
console.log(add(5, 10));
Example 3: Arrow Functions
const multiply = (x, y) => x * y;
console.log(multiply(3, 4));
6. Diving into Control Flow Statements
Control flow manages the execution of code.
If-Else Statements
if (condition) {
// Code to execute if true
} else {
// Code to execute if false
}
Example 1:
let score = 85;
if (score > 90) {
console.log("Excellent");
} else {
console.log("Good");
}
7. Exploring Objects and Arrays
Objects
Objects are key-value pairs.
Example 1: Create an Object
let person = {
name: "John",
age: 30,
isEmployed: true,
};
console.log(person.name);
Arrays
Arrays store multiple values.
Example 1:
let fruits = ["Apple", "Banana", "Cherry"];
console.log(fruits[1]);
8. Event Handling for Dynamic Web Pages
Events add interactivity to websites.
Example: Button Click
<button onclick="showAlert()">Click Me</button>
<script>
function showAlert() {
alert("Button clicked!");
}
</script>
9. Learning Asynchronous JavaScript: Promises and Async/Await
Asynchronous programming handles long-running tasks.
Promises
let promise = new Promise((resolve, reject) => {
resolve("Success!");
});
promise.then((message) => console.log(message));
Async/Await
async function fetchData() {
let data = await fetch("https://api.example.com");
console.log(data);
}
fetchData();
10. Building Real-World Projects
Practice is crucial for mastery. Create projects like:
- To-Do List: Manage tasks using DOM manipulation.
- Weather App: Fetch data from APIs.
- Interactive Quiz: Test users’ knowledge dynamically.
FAQs
Q1: How long does it take to learn JavaScript?
A: It depends on your dedication, but mastering the basics can take 1-2 months.
Q2: Is JavaScript beginner-friendly?
A: Yes, JavaScript’s syntax is simple, and it’s an excellent language for beginners.
Q3: Should I learn JavaScript in 2024?
A: Absolutely! It’s a versatile language with high demand in the job market.
Conclusion
Learning JavaScript in 2024 is a game-changer for anyone aspiring to enter web development. By following this guide, practicing consistently, and building projects, you’ll master JavaScript in no time. Dive in today and unlock endless possibilities in the digital world!