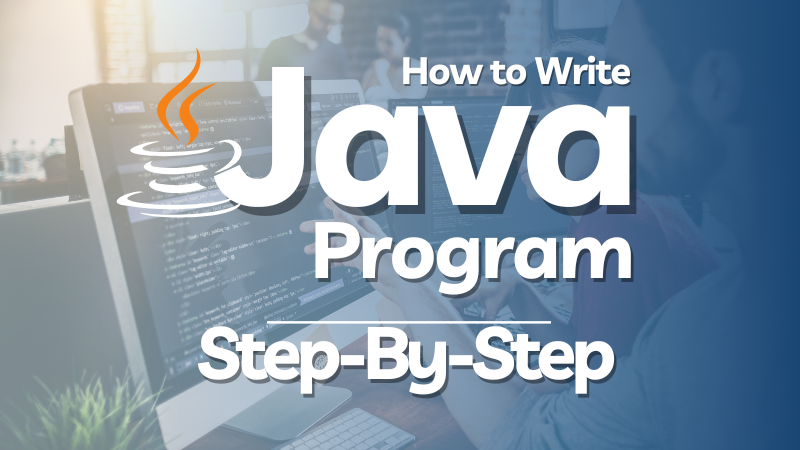
Learn how to write your first Java program with this step-by-step guide. Understand the basics of Java syntax, setup, and code structure with detailed examples and explanations.
Java is a popular, versatile programming language used in everything from web development to mobile apps. Writing your first Java program can seem daunting, but with the right guidance, it becomes manageable and rewarding. This guide provides a step-by-step approach to writing, compiling, and executing your first Java program. Along the way, we’ll explain each step in detail, including syntax, and provide multiple variations for practice.
Why Learn Java?
Java is a widely used, object-oriented programming language known for its simplicity, portability, and robustness. Here are a few reasons why learning Java can be beneficial:
- Platform Independence: Java runs on any platform that has a Java Virtual Machine (JVM).
- Versatility: Java is used in mobile development, web applications, cloud-based services, and large enterprise solutions.
- Strong Community Support: With millions of developers worldwide, Java boasts extensive community support and resources.
Setting Up Your Java Environment
Before writing your first Java program, ensure that you have Java Development Kit (JDK) installed on your machine. The JDK includes the tools necessary for compiling and running Java code.
Steps to Install Java Development Kit (JDK)
- Download the JDK:
- Visit the official Oracle Java SE download page.
- Download the latest JDK version compatible with your operating system.
- Install the JDK:
- Open the downloaded installer and follow the instructions for installation.
- Set Environment Variables (Windows only):
- Add the JDK’s bin directory to your system’s PATH environment variable so that you can access Java commands from the command line.
- Verify the Installation:
- Open a terminal (or Command Prompt in Windows) and type:bashCopy code
java -version
- If installed correctly, it should display the Java version.
- Open a terminal (or Command Prompt in Windows) and type:bashCopy code
Writing Your First Java Program
With Java set up, you’re ready to write your first program! For this guide, we’ll create a simple program that prints “Hello, World!” to the console.
Step 1: Creating a Java File
To start, open any text editor or an Integrated Development Environment (IDE) like Eclipse or IntelliJ.
- File Naming: Java files must end with
.java
(e.g.,HelloWorld.java
). - Class Name: The file name should match the class name, as Java requires a public class name to be the same as the file name.
Step 2: Writing the Code
In your file, type the following code:
// HelloWorld.java
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Code Explanation:
public class HelloWorld
: Defines a public class namedHelloWorld
.public static void main(String[] args)
: Declares the main method, which is the entry point of any Java program.System.out.println("Hello, World!");
: Prints “Hello, World!” to the console.
Step 3: Saving the File
Save the file as HelloWorld.java
in your chosen directory.
Step 4: Compiling the Java Program
To compile the Java program:
- Open your command line or terminal.
- Navigate to the directory where
HelloWorld.java
is saved. - Run the following command:bashCopy code
javac HelloWorld.java
- javac: The Java compiler, used to compile Java code into bytecode.
- If there are no errors, this command creates a
HelloWorld.class
file, which contains the bytecode for your program.
Step 5: Running the Java Program
After successful compilation, run the program with this command:
> java HelloWorld
You should see:
Hello, World!
This output confirms that your Java program is working as expected!
Understanding the Syntax of a Java Program
Every Java program has a few key components:
- Class Declaration: Every Java program must have at least one class, which serves as the blueprint for objects. The class name should match the filename.
public class HelloWorld { }
- Main Method: The
main
method is the entry point for Java applications.
public static void main(String[] args) { }
- Print Statement: The
System.out.println()
command outputs text to the console.
System.out.println("Hello, World!");
Variations in Syntax
Experimenting with different variations of the code can be helpful to better understand Java syntax.
Common Variations of Hello World Programs
Let’s explore three example variations to modify and practice the Hello World program.
Variation 1: Custom Message
Try changing the message inside System.out.println()
:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Welcome to Java Programming!");
}
}
Variation 2: Adding Multiple Print Statements
Print multiple lines by adding more System.out.println()
commands:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
System.out.println("Learning Java is fun!");
System.out.println("Let's code!");
}
}
Variation 3: Using Variables in Print Statements
Declare a variable and print it as part of the output:
public class HelloWorld {
public static void main(String[] args) {
String message = "Hello from Java!";
System.out.println(message);
}
}
Each of these variations introduces new concepts gradually, helping you build familiarity with Java syntax and programming concepts.
FAQs
1. What is the main purpose of the main method in Java?
The main method serves as the entry point for Java applications. When you run a program, the JVM looks for the main
method to start execution.
2. Why do we need to compile Java code before running it?
Compiling converts Java code into bytecode, which can then be executed by the JVM. This makes Java platform-independent.
3. Can I name my Java class anything?
The class name should match the filename, and it should be a valid identifier (starting with a letter, underscore, or dollar sign).
4. How do I run my Java program if there are multiple classes?
Only the class with the main
method is used as the entry point for execution. Compile all classes, but run the one with the main method.
5. Can I use special characters in my Java print statements?
Yes, Java supports escape sequences, like \n
for new lines and \t
for tabs, in System.out.println()
.
Conclusion
Writing your first Java program is a significant step in learning the language. By understanding the basic structure and syntax, you can start experimenting with more complex concepts in Java. Setting up your environment, writing code, compiling, and executing programs are foundational skills that will support you as you progress in Java development.
This guide has provided step-by-step instructions with example variations to reinforce your understanding. Now, you’re ready to build on this foundation, explore more advanced topics, and begin creating your own Java applications. Happy coding!