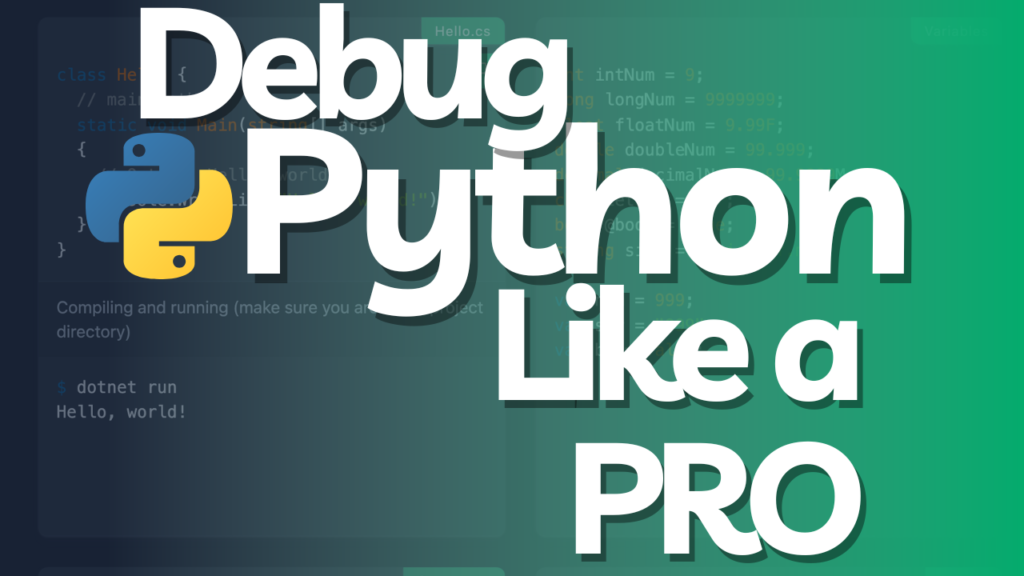
Debugging is an essential skill for every Python developer, whether you’re a beginner or seasoned programmer. Even the best-written code can encounter bugs that disrupt functionality and reduce efficiency. Fortunately, learning how to debug Python code effectively can save you time, frustration, and help you become a more skilled coder.
In this guide, we’ll explore essential debugging techniques, tools, and strategies that can elevate your Python programming skills. Let’s dive into practical, actionable ways to debug Python code like a pro!
Table of Contents
- Why Debugging Is Crucial in Python Programming
- Common Types of Bugs in Python Code
- Basic Debugging Techniques of Python Code
- Using Python’s Built-in Debugger: pdb
- Best Practices for Error Messages
- Debugging with IDE Tools
- Logging: A Pro Tool for Advanced Debugging
- Automated Testing for Preventing Bugs
- Real-Life Debugging Examples
- FAQs on Python Debugging
- Conclusion
Why Debugging Is Crucial in Python Programming
Debugging is essential to ensure that your code is accurate, efficient, and resilient. By effectively debugging Python code, you can identify issues early, enhance code performance, and avoid critical failures in production. Debugging also encourages a better understanding of how code components work together, leading to cleaner and more optimized code overall.
Common Types of Bugs in Python Code
Before we dive into debugging techniques, let’s identify the types of bugs you may encounter in Python:
1. Syntax Errors
Syntax errors occur when the code does not conform to Python’s syntax rules, often causing the program to stop running.
2. Logical Errors
Logical errors cause the program to run, but with incorrect output or behavior.
3. Runtime Errors
These errors occur during the execution of the program, often due to operations that fail at runtime, such as division by zero.
Understanding the nature of these bugs will guide you in choosing the right debugging technique to solve them effectively.
Basic Debugging Techniques of Python Code
Start with these foundational debugging techniques to tackle common Python errors:
1. Print Statements
Adding print()
statements in your code to display variable values can be a simple yet effective way to understand code flow.
2. Check for Typos
Typos in function names, variables, or keywords can lead to unexpected errors. A quick scan can sometimes resolve issues before they become complex problems.
3. Isolate the Issue
Break down your code into smaller parts to isolate the error, allowing you to focus on specific functions or loops without distraction.
4. Check for Indentation Errors
Python relies heavily on indentation. Ensuring consistent indentation helps avoid syntax errors and improves code readability.
Using these basic techniques as a first step can save time and quickly highlight issues without the need for advanced debugging tools.
Using Python’s Built-in Debugger: pdb
Python’s pdb
module is an interactive debugger that allows you to step through code, inspect variables, and analyze code behavior in real-time.
Steps to Use pdb
:
- Import
pdb
: Includeimport pdb
at the beginning of your script. - Set a Breakpoint: Insert
pdb.set_trace()
where you want the code execution to pause. - Analyze Code: Use commands like
next
to move to the next line,print
to display variable values, andcontinue
to resume execution.
Example:
import pdb
def calculate_area(radius):
area = 3.14 * (radius ** 2)
return area
pdb.set_trace() # Pauses here
result = calculate_area(5)
print(result)
Using pdb
gives you more control over debugging, especially in complex programs.
Best Practices for Error Messages
When errors arise, Python’s error messages provide valuable insight. Here’s how to make the most of them:
1. Read the Full Error Message
Python error messages indicate the type of error, location, and stack trace. Avoid skimming; each part offers clues to pinpoint the issue.
2. Trace the Stack
Follow the stack trace from the top to understand the error’s origin and any preceding operations that could have contributed to the problem.
3. Use Exception Handling to Track Errors
Catching exceptions with try-except
blocks can help manage errors without interrupting program flow.
try:
result = calculate_area(-5)
except ValueError as e:
print(f"Error encountered: {e}")
Using these practices helps you better analyze and interpret error messages, making debugging faster and more effective.
Debugging with IDE Tools
Many Python IDEs come equipped with debugging tools that simplify the debugging process. Here are a few popular ones:
1. Visual Studio Code
VS Code’s debugging tool allows breakpoints, variable inspection, and conditional debugging, making it an ideal tool for in-depth debugging.
2. PyCharm
PyCharm’s debugger supports advanced features like step-over, step-into, and run-to-cursor. You can even watch specific expressions, helping to pinpoint errors quickly.
3. Jupyter Notebook
Jupyter’s %debug
magic command provides an interactive debugging environment that is perfect for data analysis projects.
Utilizing these tools effectively can save time and increase productivity in debugging sessions.
Logging: A Pro Tool for Advanced Debugging
Logging is an advanced debugging technique that lets you monitor code behavior over time. Instead of using print()
statements, you can record information about program execution, errors, and variable states.
Setting Up Logging:
import logging
logging.basicConfig(level=logging.DEBUG)
logger = logging.getLogger(__name__)
def calculate_area(radius):
logger.debug(f"Calculating area for radius: {radius}")
if radius < 0:
logger.error("Radius cannot be negative.")
return None
return 3.14 * (radius ** 2)
Benefits of Logging:
- Detailed Information: Logs allow you to collect more details than print statements.
- File Storage: Log messages can be saved to files, enabling analysis of code execution over time.
- Custom Levels: Logging levels (
DEBUG
,INFO
,WARNING
,ERROR
,CRITICAL
) let you control the verbosity of output.
Automated Testing for Preventing Bugs
Automated testing allows you to identify bugs before your code is even deployed, providing confidence in its correctness.
Unit Testing with unittest
Python’s unittest
framework enables you to test individual units of your code.
import unittest
class TestCalculateArea(unittest.TestCase):
def test_positive_radius(self):
self.assertEqual(calculate_area(5), 78.5)
def test_negative_radius(self):
self.assertIsNone(calculate_area(-1))
if __name__ == "__main__":
unittest.main()
Integrating automated tests into your workflow is a proactive way to prevent bugs from surfacing later.
Real-Life Debugging Examples
Example 1: Debugging a Loop
for i in range(10):
print("Loop iteration:", i)
if i == 5:
break
Example 2: Debugging Function Logic
def multiply(a, b):
result = a * b
return result
print(multiply(5, '3')) # Results in a TypeError
FAQs on Python Debugging
Q1: How do I debug Python code effectively?
A: Start with basic techniques like print statements, then move to more advanced tools such as pdb
, IDE debuggers, and logging.
Q2: Can I debug Python without an IDE?
A: Absolutely! The pdb
module provides a robust debugging environment in the terminal.
Q3: What is the difference between print()
and logging?
A: While print()
is suitable for simple debugging, logging provides more control, flexibility, and is ideal for monitoring long-running applications.
Q4: How can I prevent bugs in my Python code?
A: Writing clean, modular code, using automated tests, and analyzing error messages carefully can prevent many common bugs.
Q5: How does automated testing help in debugging?
A: Automated tests catch errors early, allowing you to address potential issues before the code is deployed.
Conclusion
Debugging Python code effectively is a skill that takes time to develop but is crucial for high-quality programming. By using print statements, pdb
, IDE tools, logging, and automated tests, you can find and fix errors efficiently. As you gain experience, debugging will become a