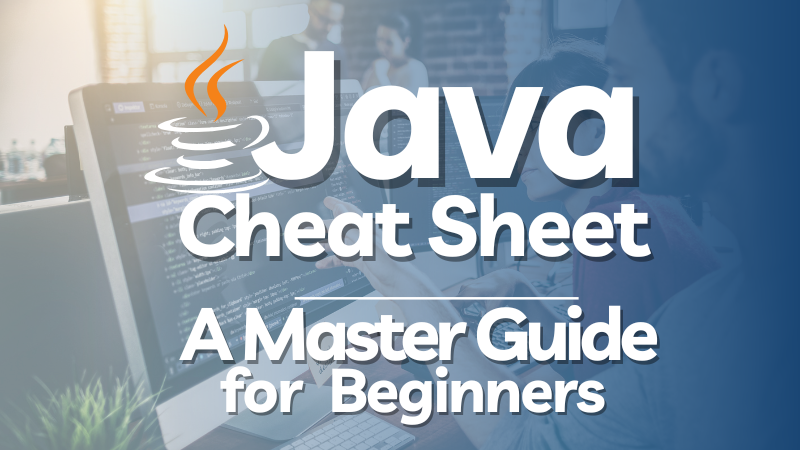
HI! Let me teach you the quickest way to Learn Java Programming, by taking your through all major concepts of Java in Cheat Sheet that focuses primarily on all basic concepts of java.
Table of Contents
Java Intro to the Course
Welcome to “Java Programming Cheat Sheet”! In this course, we’ll cover the basics of Java programming. By the end, you’ll have a foundational understanding of Java, and you’ll be able to write simple Java programs. Let’s get started!
Java Get Started with JDK and VSCode
To start programming in Java, you need to install the Java Development Kit (JDK) and an Integrated Development Environment (IDE) like Visual Studio Code (VSCode).
- Download and install the JDK from the Oracle website.
- Install VSCode from the official website.
- Configure VSCode for Java development by installing the Java Extension Pack from the Extensions Marketplace.
Java Syntax
Java syntax refers to the set of rules defining how a Java program is written. Every Java program starts with a class definition and a main method.
public class Main {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Java Output
To output text to the console, use the System.out.println method.
System.out.println("This is Java output!");
Java Comments
Comments are used to explain code and are ignored by the compiler.
// This is a single-line comment
/* This is a
multi-line comment */
Java Variables
Variables store data that can be used and manipulated in your program.
Print Variables
int myNumber = 5;
System.out.println(myNumber);
Multiple Variables
int x = 5, y = 10, z = 15;
System.out.println(x + y + z);
Java Data Types
Data types specify the type of data a variable can hold.
Java Data Types
Primitive data types include int, char, boolean, etc.
Numbers Data Type
int number = 10;
double decimal = 10.5;
Booleans Data Type
boolean isJavaFun = true;
Characters Data Type
char letter = ‘A’;
Java Type Casting
Type casting is converting one data type into another.
int myInt = 9;
double myDouble = myInt; // Automatic casting
double myDouble = 9.78;
int myInt = (int) myDouble; // Manual casting
Java Operators
Operators are used to perform operations on variables and values.
int sum = 5 + 3;
int product = 5 * 3;
Java Strings
Strings are objects that represent sequences of characters.
String greeting = “Hello, World!”;
System.out.println(greeting);
Java Math
Java provides the Math class for performing mathematical operations.
int max = Math.max(5, 10);
double sqrt = Math.sqrt(64);
Java Booleans
Booleans represent true/false values.
boolean isJavaFun = true;
boolean isFishTasty = false;
Java Conditional & Loop Statements
Conditional statements in Java allow us to make decision and add logic to our code.
Java If…Else
Conditional statements execute code based on conditions.
int time = 20;
if (time < 18) {
System.out.println("Good day.");
} else {
System.out.println("Good evening.");
}
Java Switch
Switch statements select one of many code blocks to execute.
int day = 4;
switch (day) {
case 1:
System.out.println("Monday");
break;
case 2:
System.out.println("Tuesday");
break;
case 3:
System.out.println("Wednesday");
break;
case 4:
System.out.println("Thursday");
break;
default:
System.out.println("Weekend");
}
Java While Loop
while loops execute a block of code as long as a condition is true.
int i = 0;
while (i < 5) {
System.out.println(i);
i++;
}
Java Do-While Loop
do-while loops execute a block of code once, before checking if the condition is true.
int i = 0;
do {
System.out.println(i);
i++;
} while (i < 5);
Java For Loop
for loops are used when you know in advance how many times you want to execute a statement or a block of statements.
for (int i = 0; i < 5; i++) {
System.out.println(i);
}
Java Nested Loops
Nested loops are loops inside another loop.
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
System.out.println(i + ", " + j);
}
}
Java Break/Continue
break exits the loop, and continue skips the current iteration.
for (int i = 0; i < 10; i++) {
if (i == 4) {
break;
}
if (i == 2) {
continue;
}
System.out.println(i);
}
Java Arrays
Arrays store multiple values in a single variable.
java
String[] cars = {“Volvo”, “BMW”, “Ford”};
System.out.println(cars[0]);
Java Classes, Objects and Methods
The most important aspect of Java Programming is to understand how to use Classes, Objects and Methods.
Java Methods
Methods are blocks of code that perform a specific task and can be called upon when needed.
java
public class Main {
static void myMethod() {
System.out.println(“Hello from myMethod!”);
}
public static void main(String[] args) {
myMethod(); // Calling the method
}
}
Java Method Parameters
Methods can accept parameters, which are values passed to the method.
java
public class Main {
static void myMethod(String fname) {
System.out.println(fname + ” Doe”);
}
public static void main(String[] args) {
myMethod(“John”);
myMethod(“Jane”);
}
}
Java Method Overloading
Method overloading allows a class to have more than one method with the same name, as long as their parameter lists are different.
java
public class Main {
static int plusMethod(int x, int y) {
return x + y;
}
static double plusMethod(double x, double y) {
return x + y;
}
public static void main(String[] args) {
int intResult = plusMethod(8, 5);
double doubleResult = plusMethod(4.3, 6.26);
System.out.println(“Int: ” + intResult);
System.out.println(“Double: ” + doubleResult);
}
}
Java Classes
Classes are templates for creating objects. They encapsulate data and methods that operate on the data.
java
public class Main {
int x = 5; // Attribute
public static void main(String[] args) {
Main myObj = new Main(); // Create an object of class Main
System.out.println(myObj.x); // Access attribute
}
}
Java OOP Concepts
Java follows Object-Oriented Programming (OOP) principles: Encapsulation, Inheritance, Polymorphism, and Abstraction.
Java Classes/Objects
A class is a blueprint for objects, and an object is an instance of a class.
java
public class Car {
String model = “Mustang”;
int year = 1969;
}
public class Main {
public static void main(String[] args) {
Car myCar = new Car();
System.out.println(myCar.model + ” ” + myCar.year);
}
}
Java Class Attributes / Class Variables
Class attributes (or variables) are fields inside a class.
java
public class Car {
String model;
int year;
}
Java Class Methods
Class methods are functions defined inside a class.
java
public class Car {
public void fullThrottle() {
System.out.println(“The car is going as fast as it can!”);
}
}
public class Main {
public static void main(String[] args) {
Car myCar = new Car();
myCar.fullThrottle();
}
}
Java Constructors
Constructors are special methods invoked when an object is created.
java
public class Car {
String model;
int year;
public Car(String model, int year) {
this.model = model;
this.year = year;
}
}
public class Main {
public static void main(String[] args) {
Car myCar = new Car(“Mustang”, 1969);
System.out.println(myCar.model + ” ” + myCar.year);
}
}
Java Modifiers
Modifiers are keywords that change the accessibility of classes, methods, and attributes.
- public: Accessible from any other class.
- private: Accessible only within the defined class.
- protected: Accessible within the same package and subclasses.
Java Encapsulation
Encapsulation means keeping the internal details of an object hidden from the outside world.
java
public class Person {
private String name;
// Getter
public String getName() {
return name;
}
// Setter
public void setName(String newName) {
this.name = newName;
}
}
Java Packages / API
Packages are used to group related classes. Java’s API provides pre-built classes and interfaces.
java
import java.util.Scanner; // Importing the Scanner class from java.util package
public class Main {
public static void main(String[] args) {
Scanner myObj = new Scanner(System.in);
System.out.println(“Enter username”);
String userName = myObj.nextLine();
System.out.println(“Username is: ” + userName);
}
}
Java Inheritance
Inheritance allows one class to inherit attributes and methods from another class.
java
class Vehicle {
protected String brand = “Ford”;
public void honk() {
System.out.println(“Beep, beep!”);
}
}
class Car extends Vehicle {
private String modelName = “Mustang”;
public static void main(String[] args) {
Car myCar = new Car();
myCar.honk();
System.out.println(myCar.brand + ” ” + myCar.modelName);
}
}
Java Polymorphism
Polymorphism allows methods to do different things based on the object it is acting upon.
java
class Animal {
public void animalSound() {
System.out.println(“The animal makes a sound”);
}
}
class Dog extends Animal {
public void animalSound() {
System.out.println(“The dog says: bow wow”);
}
}
public class Main {
public static void main(String[] args) {
Animal myAnimal = new Animal();
Animal myDog = new Dog();
myAnimal.animalSound();
myDog.animalSound();
}
}
Java Inner Classes
Inner classes are nested within other classes.
java
public class OuterClass {
int x = 10;
class InnerClass {
int y = 5;
}
}
public class Main {
public static void main(String[] args) {
OuterClass myOuter = new OuterClass();
OuterClass.InnerClass myInner = myOuter.new InnerClass();
System.out.println(myInner.y + myOuter.x);
}
}