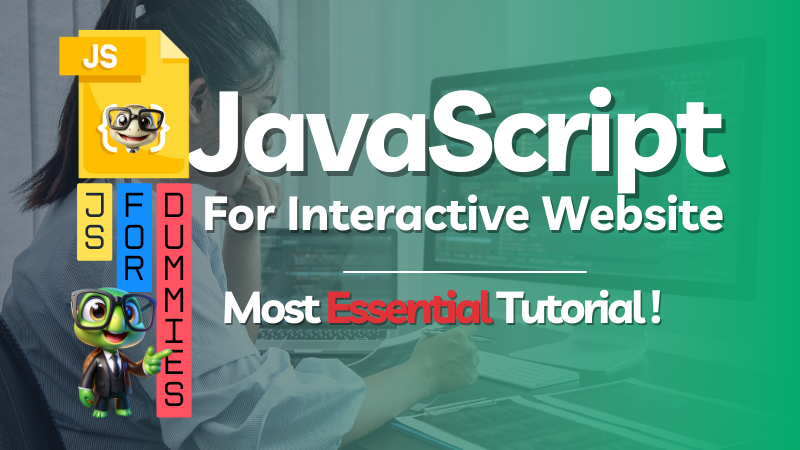
Discover how to use JavaScript to make your website interactive! This step-by-step tutorial explains the syntax, provides examples, and shares tips to create engaging, dynamic websites. Perfect for beginners and pros alike.
In today’s web development world, creating a static website is no longer enough. Users expect interactivity, seamless experiences, and responsive designs. JavaScript, a versatile and powerful scripting language, allows developers to breathe life into websites. From form validation to animations and dynamic content updates, JavaScript enables developers to create user-friendly, engaging web experiences.
In this tutorial, we’ll break down JavaScript essentials, guide you through key concepts, and provide example variations to help you implement interactivity in your projects.
Why JavaScript is Essential for Web Interactivity
JavaScript plays a pivotal role in web development because:
- Real-Time Updates: Modify website content dynamically without refreshing the page.
- Enhanced User Experience: Add interactive features like dropdown menus, sliders, and tooltips.
- Cross-Platform Compatibility: Runs on virtually all modern web browsers without additional installations.
By learning JavaScript, you can elevate your website’s functionality and provide users with a memorable experience.
Getting Started with JavaScript
To begin coding in JavaScript, you’ll need:
- A Text Editor: Use tools like Visual Studio Code, Sublime Text, or Notepad++.
- A Browser: Modern browsers like Chrome or Firefox include developer tools to debug your code.
Steps to Add JavaScript
1. Inline Script:
Place the script directly within an HTML element.
<button onclick="alert('Hello, World!')">Click Me</button>
2. Internal Script:
Embed JavaScript code within the <script>
tags inside the HTML file.
<script> console.log('Hello, Console!'); </script>
3. External Script:
Link a .js
file to your HTML.
<script src="script.js"></script>
JavaScript Syntax Basics
Understanding JavaScript syntax is crucial for writing clean, error-free code.
Variables
Variables store data for later use.
- Using
var
(Old Method):var name = "John"; console.log(name);
- Using
let
(Block Scoped):let age = 25; console.log(age);
- Using
const
(Immutable):const country = "USA"; console.log(country);
Functions
Functions encapsulate reusable code.
1. Regular Function:
function greet() { console.log("Hello, World!"); } greet();
2. Function with Parameters:
function add(a, b) { return a + b; } console.log(add(5, 3));
3. Arrow Function:
const multiply = (x, y) => x * y; console.log(multiply(4, 2));
Adding Interactivity to a Button
Adding click events to buttons is one of the simplest ways to make a website interactive.
Example 1: Displaying a Message
document.getElementById("btn").onclick = function() {
alert("Button clicked!");
};
Example 2: Changing Button Text
document.getElementById("btn").onclick = function() {
this.textContent = "Clicked!";
};
Example 3: Styling on Click
document.getElementById("btn").onclick = function() {
this.style.backgroundColor = "blue";
};
Manipulating HTML and CSS
JavaScript can dynamically alter HTML content and CSS properties.
Changing Text Content
document.getElementById("header").textContent = "Welcome to JavaScript!";
Adding CSS Styles
document.getElementById("header").style.color = "green";
Hiding/Showing Elements
document.getElementById("header").style.display = "none";
Form Validation Using JavaScript
Ensure user inputs meet requirements before submission.
Example 1: Checking Empty Fields
function validateForm() {
let name = document.forms["myForm"]["name"].value;
if (name == "") {
alert("Name must be filled out");
return false;
}
}
Example 2: Email Validation
function validateEmail(email) {
const regex = /^\S+@\S+\.\S+$/;
return regex.test(email);
}
console.log(validateEmail("test@example.com")); // true
Event Listeners – Making Your Website Responsive
Event listeners add responsiveness to your site.
Example 1: Mouse Hover Event
document.getElementById("hoverElement").addEventListener("mouseover", function() {
this.style.color = "red";
});
Example 2: Keypress Event
document.addEventListener("keydown", function(event) {
console.log("Key pressed: " + event.key);
});
Creating Animations with JavaScript
Animations enhance user engagement.
Example: Smooth Element Movement
function moveElement() {
let pos = 0;
const elem = document.getElementById("animate");
const id = setInterval(frame, 10);
function frame() {
if (pos == 350) {
clearInterval(id);
} else {
pos++;
elem.style.left = pos + "px";
}
}
}
FAQs
1. What is JavaScript used for?
JavaScript is primarily used to create dynamic and interactive web applications. It handles tasks like content updates, animations, and user inputs.
2. Can JavaScript run without a web browser?
Yes, JavaScript can run outside the browser using environments like Node.js, which is commonly used for backend development.
3. How can I debug JavaScript code?
You can use browser developer tools (F12), which provide a console and debugger for identifying issues in your JavaScript code.
This guide offers you the tools and understanding to make your website truly interactive using JavaScript. Experiment with the examples, tweak the code, and unlock your creativity. Let JavaScript transform your web projects into dynamic experiences!