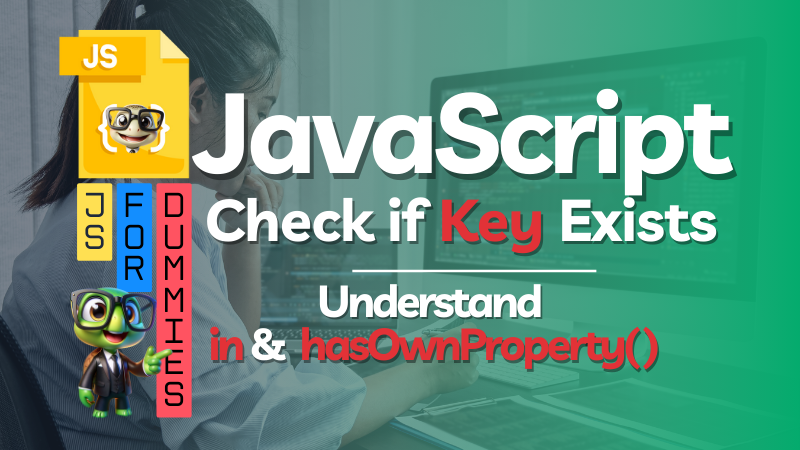
Learn how to reliably check if a JavaScript key exists in JavaScript objects or arrays. Discover the differences between in
operator, hasOwnProperty
, and other methods with detailed explanations and examples. A must-read for JavaScript developers of all levels!
In JavaScript, objects are everywhere. They’re the backbone of modern programming, storing data in JavaScript key-value pairs. Whether you’re a beginner or an experienced developer, one common question arises: “How do I check if a key exists in an object or an array?” This question might seem straightforward, but there are nuances you need to understand to ensure your code is efficient and bug-free.
In this blog, we’ll dive deep into the various methods to check for key existence in JavaScript objects and arrays. We’ll compare their pros and cons, and explore common pitfalls to avoid.
Why Key Checking is Important
Key checking is crucial because it ensures your program doesn’t encounter unexpected errors when accessing non-existent properties. For example:
- Preventing undefined values from causing bugs.
- Writing code that handles inherited properties properly.
- Debugging nested data structures efficiently.
Understanding JavaScript Objects and Arrays
In JavaScript, objects store data in the form of key-value pairs. Arrays, while being a type of object, use numerical indices as keys.
Object Example:
const obj = { name: "Alice", age: 25 };
Array Example:
const arr = [10, 20, 30];
In both cases, we often need to confirm if a specific key or index exists before proceeding.
The in
Operator
The in
operator checks if a key exists in an object, including properties inherited from its prototype chain.
Syntax:
"key" in object;
Example 1: Basic Usage
const obj = { name: "Alice", age: undefined };
console.log("name" in obj); // Output: true
console.log("salary" in obj); // Output: false
Example 2: Arrays and Indices
const arr = [10, 20, 30];
console.log(0 in arr); // Output: true
console.log(3 in arr); // Output: false
Example 3: Inherited Properties
const parentObj = { name: "Parent" };
const childObj = Object.create(parentObj);
console.log("name" in childObj); // Output: true
Key Takeaway: Use the in
operator when you need to check for both own and inherited properties.
The hasOwnProperty()
Method
The hasOwnProperty()
method checks if a key exists as a direct property of the object, ignoring inherited properties.
Syntax:
object.hasOwnProperty("key");
Example 1: Basic Usage
const obj = { name: "Alice", age: undefined };
console.log(obj.hasOwnProperty("name")); // Output: true
console.log(obj.hasOwnProperty("salary")); // Output: false
Example 2: Ignoring Inherited Properties
const parentObj = { name: "Parent" };
const childObj = Object.create(parentObj);
console.log(childObj.hasOwnProperty("name")); // Output: false
Example 3: Nested Objects
const obj = { details: { age: 25 } };
console.log(obj.hasOwnProperty("details")); // Output: true
console.log(obj.details.hasOwnProperty("age")); // Output: true
Key Takeaway: Use hasOwnProperty()
when you only want to check direct properties of an object.
Direct Access and Its Limitations
Directly accessing a key in an object returns undefined
if the key doesn’t exist. However, this can cause confusion when the key exists but its value is undefined
.
Example:
const obj = { name: "Alice", age: undefined };
console.log(obj.age); // Output: undefined
console.log(obj.salary); // Output: undefined
Why It’s Problematic:
In both cases, the output is undefined
, making it hard to differentiate between a missing key and a key with an undefined
value.
Common Mistakes and How to Avoid Them
Mistake 1: Checking for undefined
if (obj.key !== undefined) {
// Might fail if the key exists but the value is undefined.
}
Solution: Use in
or hasOwnProperty()
.
Mistake 2: Ignoring Prototype Chain
Inherited properties might lead to false positives when using in
.
Solution: Use hasOwnProperty()
if you want to ignore inherited properties.
Best Practices for Key Checking
- Use
in
for Comprehensive Checks: Covers own and inherited properties. - Use
hasOwnProperty()
for Direct Properties: Focuses on the object’s own keys. - Avoid Direct Access: Prevent ambiguity with
undefined
values. - For Arrays, Check Indices Explicitly:javascriptCopy code
const arr = [10, 20, 30]; console.log(0 in arr); // Output: true
FAQs
Q1: How do I check if a key exists in an object?
Use the in
operator or hasOwnProperty()
method for reliable checks.
Q2: What if the value of the key is undefined
?
The in
operator and hasOwnProperty()
distinguish between a missing key and a key with undefined
value.
Q3: Can I use direct access?
Direct access (obj.key
) isn’t reliable as it returns undefined
for both missing keys and keys with undefined
values.
Q4: What’s the difference between in
and hasOwnProperty()
?
The in
operator checks both own and inherited properties, while hasOwnProperty()
only checks the object’s own properties.
Conclusion
Checking if a key exists in a JavaScript object or array is a fundamental skill for developers. By understanding the nuances of the in
operator and hasOwnProperty()
, you can write more reliable and maintainable code.
Use the right method based on your requirements, and always test edge cases for robustness. If you found this article helpful, share it with fellow developers! Happy coding!