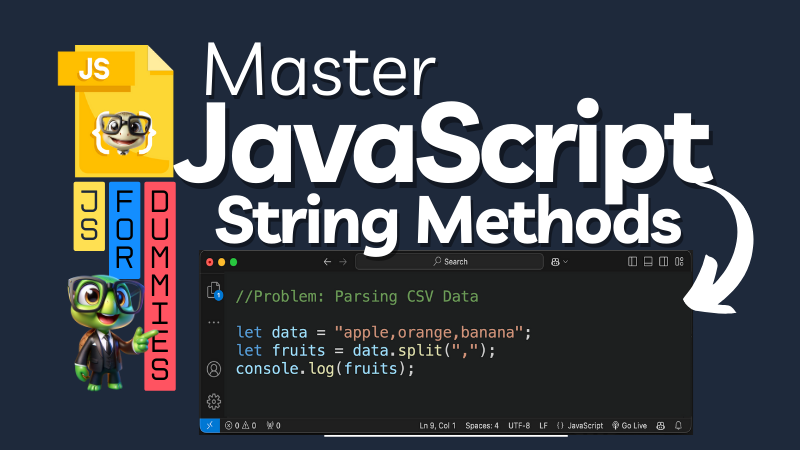
Discover the ultimate guide to JavaScript string methods! Learn powerful techniques to manipulate, extract, and transform strings effortlessly. Perfect for beginners and experts alike.
Introduction
In the dynamic world of web development, JavaScript string methods play a crucial role in transforming raw text into meaningful data. Whether you’re building a dynamic webpage or parsing user input, mastering these methods can significantly enhance your coding efficiency and performance.
This guide reveals the secret behind JavaScript string methods, showcasing their applications, examples, and tips for best practices. Let’s dive deep into the strings of JavaScript and unlock their true potential!
Why Are String Methods Important in JavaScript?
Strings are one of the most common data types in JavaScript. They are used to display content, process data, and communicate between servers and clients. String methods:
- Simplify text manipulation.
- Enable powerful operations like search and formatting.
- Improve code readability and maintainability.
Understanding JavaScript String
In JavaScript, a string is a sequence of characters enclosed in quotes (' '
, " "
, or backticks `
). Here’s an example:
let message = "Hello, JavaScript!";
Strings can represent anything from a single word to large chunks of text and are essential for web development tasks like generating dynamic HTML, validating inputs, or storing JSON data.
Top JavaScript String Methods
1. .length
– Measure String Length
The .length
property returns the number of characters in a string, including spaces.
let text = "Learn JavaScript!";
console.log(text.length); // Output: 17
Use Case: Measuring input fields in form validation.
2. .toUpperCase()
and .toLowerCase()
– Change Case
Convert a string to uppercase or lowercase for uniformity.
let name = "JavaScript";
console.log(name.toUpperCase()); // Output: JAVASCRIPT
console.log(name.toLowerCase()); // Output: javascript
Use Case: Normalizing data for comparison.
3. .trim()
– Remove Whitespace
Eliminates extra spaces from both ends of a string.
let greeting = " Hello! ";
console.log(greeting.trim()); // Output: "Hello!"
Use Case: Cleaning user input.
4. .slice()
– Extract a Portion of a String
Extracts a specific section of a string based on start and end indices.
let phrase = "JavaScript is fun!";
console.log(phrase.slice(0, 10)); // Output: JavaScript
Use Case: Displaying previews or summaries.
5. .substring()
– Another Way to Extract Substrings
Similar to .slice()
, but does not accept negative indices.
console.log(phrase.substring(0, 10)); // Output: JavaScript
6. .replace()
and .replaceAll()
– Replace Content
Replace parts of a string with new content.
let sentence = "I love JavaScript.";
console.log(sentence.replace("love", "adore")); // Output: I adore JavaScript.
// Using replaceAll
let data = "apple, apple, apple";
console.log(data.replaceAll("apple", "orange")); // Output: orange, orange, orange
Use Case: Updating templates or placeholders dynamically.
7. .includes()
and .indexOf()
– Search for Substrings
Check if a string contains specific text or find its position.
let text = "Learning JavaScript";
console.log(text.includes("JavaScript")); // Output: true
console.log(text.indexOf("JavaScript")); // Output: 9
Use Case: Implementing search features.
8. .split()
– Divide a String
Break a string into an array of substrings based on a delimiter.
let tags = "HTML,CSS,JavaScript";
console.log(tags.split(",")); // Output: ['HTML', 'CSS', 'JavaScript']
Use Case: Processing CSV data.
9. .concat()
– Merge Strings
Combine two or more strings.
let firstName = "John";
let lastName = "Doe";
console.log(firstName.concat(" ", lastName)); // Output: John Doe
10. .charAt()
and .charCodeAt()
– Character Details
Retrieve a specific character or its Unicode value.
let word = "JavaScript";
console.log(word.charAt(0)); // Output: J
console.log(word.charCodeAt(0)); // Output: 74
Use Case: Handling text-based encryption or formatting.
Common Use Cases for JavaScript String Methods
- Form Validation: Ensure proper formatting of names, emails, and passwords.
- Data Parsing: Extract and process data from files or APIs.
- User Experience: Enhance search features with
.includes()
or auto-complete suggestions.
Advanced Tips for JavaScript String Manipulation
- Optimize Performance: Minimize chaining multiple methods for better efficiency.
- Regular Expressions: Combine
.replace()
with regex for powerful text transformations. - Template Literals: Use backticks for dynamic string construction.
Example:
let user = "Alice";
let score = 95;
console.log(`Congratulations, ${user}! Your score is ${score}.`);
FAQs About JavaScript String Methods
1. What are JavaScript string methods used for?
String methods manipulate and extract information from strings, making text processing easy and efficient.
2. Can string methods modify the original string?
No, string methods do not alter the original string as strings are immutable in JavaScript.
3. How do I handle large text files in JavaScript?
Use .split()
to break content into manageable chunks and .trim()
to clean unnecessary spaces.
4. What’s the difference between .slice()
and .substring()
?
.slice()
supports negative indices; .substring()
does not.
Conclusion
Mastering JavaScript string methods is essential for any developer aiming to build robust and efficient applications. By leveraging these methods, you can simplify complex text operations, improve code clarity, and enhance your web projects.
Ready to unlock the secrets of JavaScript strings? Start experimenting today!