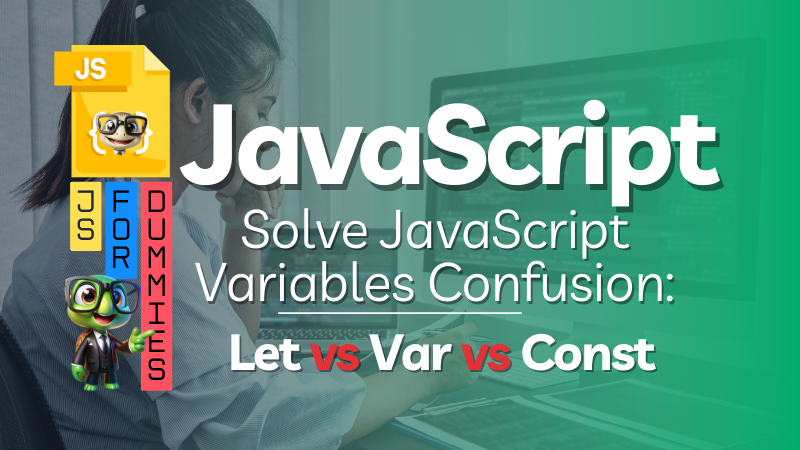
Learn how to eliminate confusion about JavaScript variables. Dive deep into var
, let
, and const
with practical examples, clear explanations, and essential tips for better coding practices.
Introduction
Understanding variables in JavaScript is critical for any developer. Yet, concepts like var
, let
, and const
often leave beginners scratching their heads. Why do we need three different ways to declare variables? When should we use one over the others? This article takes a deep dive into JavaScript variables to clear up the confusion once and for all.
Whether you’re a beginner or an experienced developer, mastering variables will help you write cleaner, more efficient code. Let’s break it all down step by step.
What Are Variables in JavaScript?
Variables are containers for storing data values. They allow you to label data and use it throughout your program. Think of variables as named storage boxes where you can place values like numbers, strings, or objects.
In JavaScript, variables are declared using the keywords var
, let
, or const
. The choice of keyword determines how the variable behaves in terms of scope, reusability, and immutability.
Introduction to var
, let
, and const
Overview of Each Keyword
var
: The original way to declare variables in JavaScript, introduced in ES5 and earlier.let
: Introduced in ES6 to address shortcomings ofvar
, offering block-scoped variables.const
: Also introduced in ES6, used for variables whose value should not change.
Why Three Keywords?
JavaScript evolved over time. The introduction of let
and const
in ES6 aimed to make code more predictable and reduce errors. Each serves a specific purpose depending on the requirements of your code.
Understanding var
: The Old Way
Before ES6, var
was the only way to declare variables. Here’s what you need to know:
Characteristics of var
:
- Function Scope: Variables declared with
var
are accessible throughout the function in which they are declared. - Hoisting:
var
declarations are “hoisted” to the top of their scope, meaning they can be used before their declaration. - Re-declaration Allowed: You can declare the same
var
variable multiple times without an error.
Example:
function demoVar() {
console.log(x); // undefined due to hoisting
var x = 5;
console.log(x); // 5
}
demoVar();
While functional, var
often led to bugs due to hoisting and a lack of block-level scoping.
Modern Declarations with let
With the introduction of let
in ES6, JavaScript gained more reliable variable handling.
Characteristics of let
:
- Block Scope: Variables declared with
let
are only accessible within the block they are declared in. - No Hoisting in Practice: While technically hoisted, accessing
let
variables before declaration results in aReferenceError
. - Re-declaration Not Allowed: Unlike
var
,let
prevents re-declaration in the same scope.
Example:
function demoLet() {
if (true) {
let x = 10;
console.log(x); // 10
}
// console.log(x); // Error: x is not defined
}
demoLet();
let
provides better control, especially in loops and conditional blocks.
Immutability with const
const
is your go-to for declaring variables that shouldn’t change.
Characteristics of const
:
- Block Scope: Like
let
,const
is block-scoped. - Immutability: Variables declared with
const
cannot be reassigned. - Hoisting Rules: Similar to
let
, accessingconst
before declaration results in aReferenceError
.
Example:
const PI = 3.14;
// PI = 3.14159; // Error: Assignment to constant variable
Exception: Mutable Objects
While the reference to a const
variable cannot change, the contents of objects or arrays can be modified.
const arr = [1, 2, 3];
arr.push(4); // Works!
console.log(arr); // [1, 2, 3, 4]
Key Differences Between var
, let
, and const
Feature | var | let | const |
---|---|---|---|
Scope | Function | Block | Block |
Re-declaration | Allowed | Not Allowed | Not Allowed |
Re-assignment | Allowed | Allowed | Not Allowed |
Hoisting | Yes | Yes (Temporal Dead Zone) | Yes (Temporal Dead Zone) |
Scope and Hoisting Explained
Scope:
- Global Scope: Variables accessible anywhere in the program.
- Function Scope: Variables accessible only within a function.
- Block Scope: Variables accessible only within the enclosing
{}
.
Hoisting:
Hoisting refers to how JavaScript moves variable and function declarations to the top of their scope during compile time.
Best Practices for Variable Declaration
- Default to
const
: Useconst
unless you need to reassign the variable. - Use
let
for Loops: For variables that need to change within a loop or block, uselet
. - Avoid
var
: Stick tolet
andconst
for more predictable behavior.
Common Pitfalls and How to Avoid Them
- Misunderstanding Hoisting: Always declare variables at the top of their scope.
- Using
var
in Loops: Leads to unexpected results due to function scope. Uselet
instead. - Overusing Global Variables: Limit global scope to avoid conflicts and bugs.
FAQs About JavaScript Variables
1. What is the best way to declare variables in JavaScript?
Use const
by default. Switch to let
only when re-assignment is necessary. Avoid var
.
2. Can I reassign an object declared with const
?
No, but you can modify its properties.
3. Why does var
lead to bugs?
Because it’s function-scoped and hoisted, leading to unintended behavior in blocks.
4. What is the Temporal Dead Zone?
It’s the period between entering a block and the variable being declared where accessing let
or const
results in an error.
5. Is var
completely obsolete?
Not entirely, but its use is discouraged in modern JavaScript development.
Conclusion
Solving JavaScript variable confusion starts with understanding the behavior of var
, let
, and const
. By following best practices and leveraging block-scoped declarations, you can write cleaner, more predictable code. With the insights from this guide, you’re now equipped to tackle any variable-related challenge in JavaScript.
Start coding with confidence!