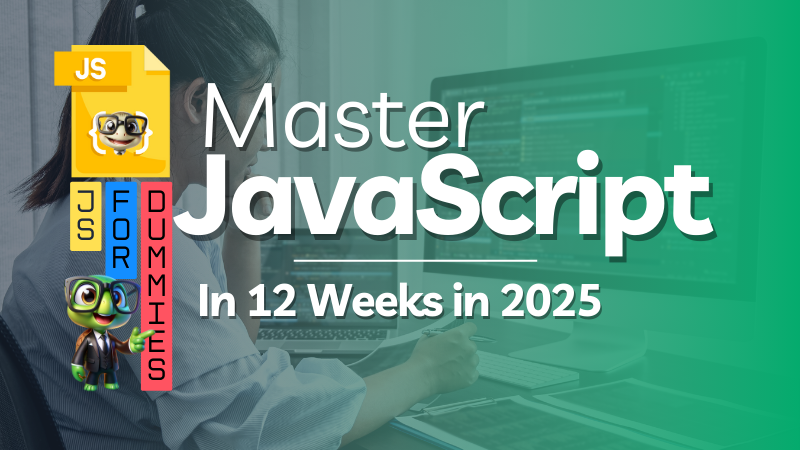
Learn how to master JavaScript in just 12 weeks with this ultimate roadmap. Follow our weekly plan to become a full-stack web developer. Perfect for beginners and aspiring coders!
Introduction: Why JavaScript Is Essential in 2024
JavaScript has become the cornerstone of modern web development. Whether you’re building dynamic front-end applications or robust back-end systems, mastering JavaScript is non-negotiable. In this comprehensive guide, we’ll walk you through a 12-week roadmap designed to help you become proficient in JavaScript and achieve your full-stack web development goals.
This guide is perfect for beginners and those looking to refine their skills. We’ll break down each week into manageable tasks, helping you stay consistent and track your progress effectively.
H1: Week 1: Getting Started with JavaScript Basics
H2: Set Up Your Environment
Before diving into coding, ensure you have the right tools:
- Code Editor: Download and install VS Code.
- Browser Tools: Familiarize yourself with Chrome Developer Tools for debugging.
- Node.js: Install Node.js for running JavaScript outside the browser.
H3: Core Concepts to Learn
- What is JavaScript? A high-level, versatile programming language primarily used for web development.
- How JavaScript Works: Learn how browsers execute JavaScript through the JavaScript engine.
- Writing Your First Program:
console.log("Hello, World!"); // Outputs "Hello, World!" in the console
Practice Tasks:
- Create a script to print your name and age.
- Write a program that calculates the sum of two numbers.
H1: Week 2: Understanding Variables and Data Types
H2: Key Topics to Master
- Variables:
let
,const
, andvar
. - Data Types: Strings, Numbers, Booleans, Arrays, and Objects.
H3: Example Code Variations
Declaring Variables
let name = "Alice"; // A variable that can be reassigned
const age = 25; // A constant variable
var isStudent = true; // An older declaration method
Practicing Data Types
// Strings
let greeting = "Hello, World!";
// Numbers
let score = 100;
// Booleans
let isLoggedIn = false;
// Arrays
let fruits = ["Apple", "Banana", "Mango"];
// Objects
let user = { name: "John", age: 30, isMember: true };
Exercises:
- Create an array of your favorite movies.
- Write an object representing a car with attributes like
brand
,model
, andyear
.
H1: Week 3: Mastering Functions and Control Flow
H2: Functions
Functions are reusable blocks of code. Understand function declaration, expression, and arrow functions.
Example Variations
// Function Declaration
function greet() {
console.log("Welcome!");
}
// Function Expression
const sum = function(a, b) {
return a + b;
};
// Arrow Function
const multiply = (x, y) => x * y;
H2: Control Flow
Master if-else
and switch
for decision-making.
// If-Else Example
if (score > 50) {
console.log("Pass");
} else {
console.log("Fail");
}
// Switch Example
let day = "Monday";
switch (day) {
case "Monday":
console.log("Start of the week");
break;
case "Friday":
console.log("End of the week");
break;
default:
console.log("Midweek");
}
Exercises:
- Write a function to check if a number is even or odd.
- Build a program to categorize ages into child, teenager, or adult.
H1: Week 4-5: Arrays and Objects
H2: Working with Arrays
Learn array methods like push
, pop
, map
, and filter
.
let numbers = [1, 2, 3, 4];
// Adding an element
numbers.push(5);
// Removing the last element
numbers.pop();
// Mapping
let squared = numbers.map(num => num * num);
// Filtering
let evenNumbers = numbers.filter(num => num % 2 === 0);
H2: Understanding Objects
Work with object properties, methods, and nested objects.
let user = {
name: "Alice",
age: 25,
greet: function() {
console.log("Hello!");
}
};
// Accessing properties
console.log(user.name);
// Calling methods
user.greet();
H1: Week 6-7: DOM Manipulation
H2: Access and Modify Elements
Learn how to dynamically update HTML content using JavaScript.
// Selecting elements
let heading = document.getElementById("title");
// Changing content
heading.innerText = "Updated Title";
H2: Handle Events
Add interactivity through event listeners.
document.getElementById("button").addEventListener("click", function() {
alert("Button clicked!");
});
Project: Build a to-do list where users can add, edit, and delete tasks.
H1: Week 8-9: Asynchronous JavaScript
H2: Promises and Async/Await
// Fetch Example
fetch("https://jsonplaceholder.typicode.com/posts")
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error("Error:", error));
// Async/Await Example
async function fetchData() {
try {
let response = await fetch("https://jsonplaceholder.typicode.com/posts");
let data = await response.json();
console.log(data);
} catch (error) {
console.error("Error:", error);
}
}
H1: Week 10-12: Frameworks and Capstone Project
H2: Learn a Front-End Framework
Start with React or Vue.js. Understand components, state, and props.
Capstone Project Ideas:
- Build a blog site with React.
- Create an e-commerce website with Node.js and Express.
FAQs
Q1: How long does it take to learn JavaScript?
It takes about 3-6 months of consistent practice to become proficient.
Q2: Can I learn JavaScript without a programming background?
Yes, JavaScript is beginner-friendly and widely supported with resources.
Q3: Should I learn JavaScript before frameworks?
Absolutely. Master the fundamentals before diving into frameworks like React.
Conclusion
By following this 12-week roadmap, you’ll build a strong foundation in JavaScript and gain the skills necessary to create dynamic web applications. Remember, consistency is key. Practice, build projects, and stay motivated. Let JavaScript unlock your web development potential in 2024!