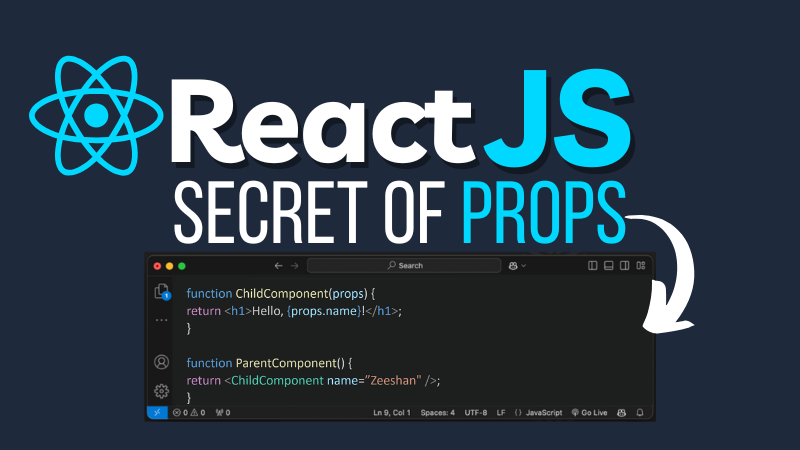
Discover the magic of passing data between components in ReactJS with this in-depth guide on props. Learn what props are, their syntax, real-world applications, and how to create reusable, dynamic components with practical examples.
Introduction to Props in ReactJS
When working with ReactJS, one of the most fundamental concepts you’ll encounter is props, short for properties. Props enable components to communicate with each other by passing data, making them dynamic and reusable. Whether you’re a beginner or already familiar with React, mastering props is crucial to building scalable, maintainable applications.
In this blog post, we’ll uncover the secret of props in ReactJS and show you how to efficiently use them for passing data between components. We’ll also provide real-world examples, syntax explanations, and FAQs to solidify your understanding.
Table of Content
What are Props in ReactJS?
Definition of Props
Props, or properties, are a mechanism to send data from a parent component to a child component in React. They act like parameters in a function, allowing you to customize how a child component behaves or what it displays.
Key Features of Props
- Read-Only: Props are immutable, meaning they cannot be modified by the receiving component.
- Unidirectional Data Flow: Props flow from parent to child components, ensuring a predictable flow of data.
- Dynamic UI: By passing different values as props, you can create components that adapt to various scenarios.
Advantages of Using Props in ReactJS
- Facilitate Component Communication: Props enable seamless data transfer between components, especially from parent to child.
- Encourage Reusability: You can design reusable components that behave differently based on the props they receive.
- Maintain Clean Code: Props help in organizing and structuring code, making it easier to read and maintain.
- Simplify Complex UIs: By breaking down a UI into smaller components and using props to communicate, you can manage complexity effectively.
How to Use Props in ReactJS?
Basic Syntax of Props
Using props involves two main steps:
- Pass Props: Define the props as attributes when using the child component in the parent.
- Access Props: Use
props
in the child component to access the passed data.
Example Code:
jsxCopy codefunction Greeting(props) {
return <h1>Hello, {props.name}!</h1>;
}
function App() {
return <Greeting name="John" />;
}
Output:
Hello, John!
Explanation:
- The parent component
<App />
sends a propname
with the value “John” to<Greeting />
. - The child component
<Greeting />
receives thename
prop and displays it.
Real-World Applications of Props in ReactJS
Props shine in real-world scenarios. Here are three practical problems and their solutions.
Problem 1: Dynamic Greeting Card
Scenario:
You’re building an application that displays personalized greeting cards. The message on each card should depend on the user’s name and favorite color.
Solution Code:
jsxCopy codefunction GreetingCard(props) {
return (
<div>
<h1>Welcome, {props.name}!</h1>
<p>Your favorite color is {props.color}.</p>
</div>
);
}
function App() {
return <GreetingCard name="Alice" color="blue" />;
}
Output:
csharpCopy codeWelcome, Alice!
Your favorite color is blue.
Explanation:
- The
GreetingCard
component receivesname
andcolor
as props and uses them to display a personalized message. - You can reuse this component for any user by changing the values of the props.
Problem 2: Handling Button Clicks with Props
Scenario:
You need a button in a child component that triggers an alert in the parent when clicked.
Solution Code:
jsxCopy codefunction Button(props) {
return <button onClick={props.handleClick}>Click Me!</button>;
}
function App() {
const showAlert = () => alert('Button clicked!');
return <Button handleClick={showAlert} />;
}
Output:
When the button is clicked, an alert pops up: Button clicked!
Explanation:
- The parent passes a
handleClick
function as a prop to theButton
component. - The
Button
component uses this function in itsonClick
event to trigger the parent’s alert.
Problem 3: Reusable Product Cards
Scenario:
Create a reusable product card component that displays different products based on the props it receives.
Solution Code:
jsxCopy codefunction ProductCard(props) {
return (
<div>
<h2>{props.productName}</h2>
<p>Price: ${props.price}</p>
<button>Add to Cart</button>
</div>
);
}
function App() {
return (
<div>
<ProductCard productName="Laptop" price="999" />
<ProductCard productName="Phone" price="499" />
</div>
);
}
Output:
Two product cards are displayed, one for a laptop and another for a phone.
Explanation:
- The
ProductCard
component is flexible and reusable. - By changing the
productName
andprice
props, you can use it for multiple products.
Common Mistakes When Using Props
- Trying to Modify Props in the Child Component:
Props are immutable. Use state if you need to modify data. - Forgetting to Pass Required Props:
Always ensure that necessary props are passed to avoid undefined errors. - Overusing Props:
If too many props are passed, consider using context or state management libraries like Redux.
FAQs
1. Can I pass functions as props?
Yes! Functions can be passed as props to trigger parent component actions from the child. This is particularly useful for handling events.
2. Are props the same as state?
No, props and state are different. Props are immutable and passed down, whereas state is mutable and managed within a component.
3. Can props be passed in both directions?
Props follow a unidirectional flow (parent to child). To send data from child to parent, you can pass a function as a prop.
Conclusion
Props in ReactJS are a game-changer when it comes to passing data between components. They make your applications dynamic, reusable, and easy to maintain. By mastering props, you’ll take your React skills to the next level, enabling you to build powerful and scalable applications.
Whether it’s creating a personalized greeting card, handling events, or building reusable components, props are your go-to tool. Start experimenting with props today and unlock the full potential of ReactJS!