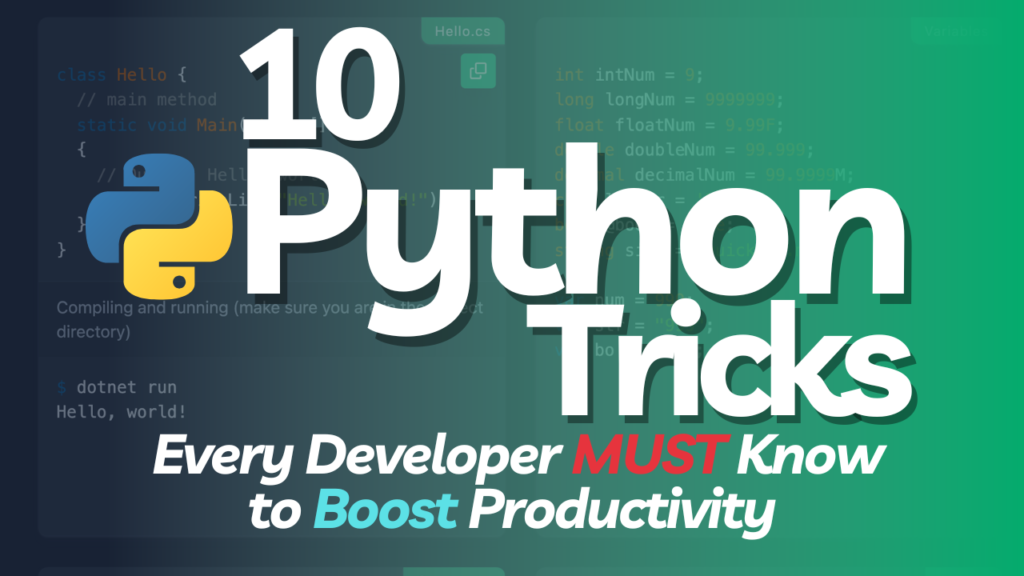
Why Python is Popular Among Developers
Python has become one of the most widely used programming languages, especially among developers who need simplicity and power combined. Known for its readable syntax and vast ecosystem, Python helps developers build solutions quickly and efficiently. But beyond its basic functionality, Python also offers numerous shortcuts and tricks that can significantly boost productivity. Let’s dive into ten Python tricks every developer should know to make their code faster, cleaner, and more effective.
1. List Comprehensions in Python
Understanding List Comprehensions and How They Work
List comprehensions offer a concise way to create lists. Instead of using traditional for loops, a single line of code can achieve the same result.
squared_numbers = [x**2 for x in range(10)]
This generates a list of squares for numbers 0 through 9.
Practical Examples of Using List Comprehensions
List comprehensions are perfect for transforming and filtering data within a list. For instance, creating a list of even numbers:
even_numbers = [x for x in range(20) if x % 2 == 0]
Using this trick reduces the lines of code and improves readability.
2. Lambda Functions in Python
When to Use Lambda Functions
Lambda functions, or anonymous functions, are useful for short-term tasks, especially when you need a quick function without formally defining it.
multiply = lambda x, y: x * y
print(multiply(2, 3)) # Outputs: 6
Common Use Cases
Lambda functions are often used in functions like map()
, filter()
, and sorted()
:
names = ["Alice", "Bob", "Charlie"]
names_sorted = sorted(names, key=lambda x: len(x))
3. Unpacking in Python
Multiple Assignments with Unpacking
Unpacking allows you to assign multiple variables in a single line:
x, y, z = [1, 2, 3]
Using Unpacking in Functions and Loops
Unpacking is especially useful in loops and functions, making it easier to handle multiple return values from functions.
4. Enumerate in Python
Why Enumerate is Better Than Traditional Loops
The enumerate()
function gives you access to both the index and the item when iterating over a list, making your code cleaner and more readable.
for index, item in enumerate(['a', 'b', 'c']):
print(index, item)
Practical Examples of Enumerate
It’s commonly used in situations where both the item and its index are required, which is frequent in data processing and analysis.
5. F-Strings in Python
Formatting Strings with F-Strings
F-strings offer a simple and efficient way to format strings, introduced in Python 3.6.
name = "Alice"
print(f"Hello, {name}!")
Tips for Using F-Strings in Complex Scenarios
F-strings can also handle expressions directly, making them highly flexible:
value = 10
print(f"The square of {value} is {value**2}")
6. Zip Function in Python
How to Use Zip to Combine Lists
The zip()
function combines elements from two or more iterables, creating pairs of corresponding elements.
list1 = [1, 2, 3]
list2 = ['a', 'b', 'c']
combined = list(zip(list1, list2))
Real-World Examples of Zip
Useful in scenarios like pairing student names with their scores, combining multiple lists becomes seamless with zip()
.
7. Dictionary Comprehensions in Python
Similarities with List Comprehensions
Dictionary comprehensions are similar to list comprehensions, providing a compact way to construct dictionaries.
squared_dict = {x: x**2 for x in range(5)}
Practical Uses for Dictionary Comprehensions
They’re ideal for cases where you need to generate dictionaries dynamically, such as creating mappings from data sets.
8. Using the ‘any’ and ‘all’ Methods in Python
Difference Between any()
and all()
any()
returnsTrue
if at least one element is true.all()
returnsTrue
if all elements are true.
numbers = [1, 2, 0, 3]
print(any(numbers)) # True
print(all(numbers)) # False
Situations Where They’re Most Useful
These functions are beneficial for checks in data validation, where you need to confirm if a condition applies to elements in a list.
9. Merging Dictionaries in Python
New Ways to Merge Dictionaries in Python
As of Python 3.9, dictionaries can be merged using the |
operator:
dict1 = {"a": 1, "b": 2}
dict2 = {"b": 3, "c": 4}
merged = dict1 | dict2
Comparison of Old and New Methods
In older Python versions, you had to use the update()
method or unpack dictionaries, but the |
operator is more elegant and readable.
10. Handling Exceptions Smartly in Python
Common Error Types and How to Handle Them
Python’s try-except
blocks allow developers to handle exceptions gracefully.
try:
result = 10 / 0
except ZeroDivisionError:
print("Cannot divide by zero")
Using ‘else’ and ‘finally’ with Try-Except Blocks
The else
block runs if no exceptions occur, and finally
always executes, ensuring proper resource management.
Conclusion:
Recap of How These Tricks Enhance Productivity
Mastering these Python tricks can save time, reduce errors, and make your code cleaner and more efficient. From list comprehensions to advanced error handling, each of these techniques contributes to a smoother development process and better productivity.
FAQs
Q1: Why should I use list comprehensions?
List comprehensions provide a concise way to create lists, making your code cleaner and often faster.
Q2: What are lambda functions used for?
Lambda functions are quick, anonymous functions useful for short tasks, especially when used with map()
and filter()
.
Q3: How does enumerate improve loops?enumerate()
allows you to access both the index and item, making code more readable when looping through lists.
Q4: What’s the advantage of f-strings over older formatting methods?
F-strings are faster and easier to read, introduced in Python 3.6, and allow expressions directly within strings.
Q5: How do any() and all() differ?any()
checks if any item is true in a list, while all()
requires all items to be true for it to return True
.
Q6: How can merging dictionaries with | operator improve my code?
This new method introduced in Python 3.9 simplifies merging dictionaries, making code more readable and concise.