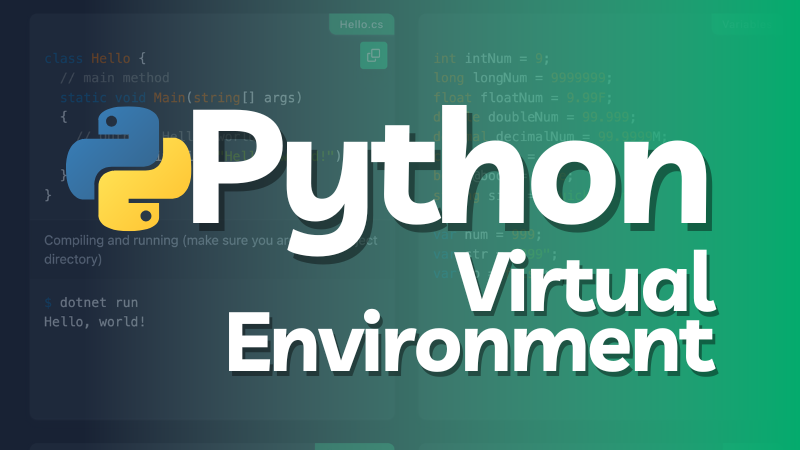
Learn how to create and use Python virtual environments to keep your projects organized, dependency-free, and error-free. Discover the benefits, setup steps, and best practices for efficient Python project management.
In Python, managing dependencies is crucial for developing robust and error-free applications. A common solution is to use virtual environments, which allow developers to separate project dependencies and avoid conflicts. This guide provides a comprehensive introduction to Python virtual environments, how to set them up, and why they’re essential for organized and maintainable project management.
What is a Python Virtual Environment?
A Python virtual environment is an isolated directory that contains its own installation of Python, along with any packages or libraries the project requires. Virtual environments separate project dependencies, allowing each project to have its own specific packages and versions without affecting other projects.
By using virtual environments, you prevent dependency conflicts and avoid the “it works on my machine” problem, making your code more portable, manageable, and less prone to unexpected behavior.
Why Use Python Virtual Environments?
Using Python virtual environments offers numerous benefits:
- Isolation: Each environment operates independently, with its own packages, so changes in one environment don’t affect others.
- Dependency Management: Easily manage and install different versions of libraries specific to each project.
- Enhanced Collaboration: Sharing environment files like
requirements.txt
makes it easier for collaborators to replicate the environment on their machines. - Consistent Environments: Virtual environments make projects more portable and ensure consistency across different machines and deployment environments.
How to Set Up a Python Virtual Environment
Setting up a virtual environment is straightforward, but the process can vary slightly depending on your Python version.
Step 1: Creating a Virtual Environment
To create a Python virtual environment, use the built-in venv
module. Here’s how to do it:
Using venv
in Python 3:
- Navigate to Your Project Directory: Open a terminal and navigate to the directory where you want to create the environment.
- Run the Command: Use the following command to create the environment:
python3 -m venv myenv
Replacemyenv
with your preferred environment name. - Directory Structure: This creates a new directory (e.g.,
myenv
) containing subdirectories for Python binaries, libraries, and scripts.
Using virtualenv
(alternative for older Python versions):
If your Python version doesn’t include the venv
module, install virtualenv
:
> pip install virtualenv
> virtualenv myenv
Step 2: Activating a Virtual Environment
To work within your virtual environment, you’ll need to activate it:
- Windows:
> myenv\Scripts\activate
- MacOS/Linux:
> source myenv/bin/activate
When activated, you should see the environment name (e.g., (myenv)
) appear in your command line prompt, indicating you’re working within the virtual environment.
Step 3: Deactivating a Virtual Environment
Once you finish working on your project, deactivate the environment by typing:
> deactivate
This returns you to your global Python environment and prevents accidental installations in the virtual environment.
Installing and Managing Packages in Virtual Environments
Once your virtual environment is activated, you can install packages specific to your project. The pip tool makes it simple:
- Installing a Package:
> pip install package_name
2. Freezing Dependencies: To create a list of installed packages and their versions, use:
> pip freeze > requirements.txt
3. Installing from Requirements File:
> pip install -r requirements.txt
These commands make it easy to share environments, as teammates can recreate the environment by installing dependencies from requirements.txt
.
Best Practices for Using Virtual Environments
To maximize the benefits of Python virtual environments, consider the following best practices:
- Use a Requirements File: Always create a
requirements.txt
file for each project. This ensures consistent dependency management across different machines. - Name Environments Consistently: Using standardized names (e.g.,
.venv
orenv
) for environments in each project can prevent confusion. - Isolate Every Project: Create a separate environment for each project to keep dependencies isolated.
- Check for Compatibility: Test package compatibility within the virtual environment to avoid dependency conflicts.
- Version Control Environment Files Only: Don’t version control the environment directory itself; instead, only include environment files (like
requirements.txt
) in version control.
Alternative Tools to Python Virtual Environments
While venv
and virtualenv
are standard tools for creating virtual environments, there are several alternatives that offer advanced features:
1. Pipenv
- Combines
pip
andvirtualenv
functionalities and uses aPipfile
to manage dependencies. - Automatically handles virtual environment creation and package installation.
2. Conda
- A package manager that can manage virtual environments and install dependencies for languages like Python and R.
- Commonly used in data science for managing packages and dependencies.
3. Poetry
- A dependency management tool that automates package installation, versioning, and virtual environment management.
- It uses a
pyproject.toml
file for configuration, simplifying the setup process.
Comparing Virtual Environment Tools
Each tool has its strengths; venv
is lightweight and part of Python, while Conda
and Pipenv
add powerful management capabilities. Choose a tool based on your project requirements and team preferences.
FAQs
1. What is the main purpose of a Python virtual environment?
Virtual environments are used to isolate dependencies between projects, ensuring that one project’s libraries don’t interfere with another’s.
2. Can I use multiple virtual environments on the same project?
Generally, one virtual environment per project is recommended to maintain clean dependency management.
3. Is it necessary to deactivate a virtual environment?
While it’s not mandatory, deactivating environments prevents accidental package installation in an active environment.
4. How do I delete a virtual environment?
Simply delete the environment directory (e.g., rm -rf myenv
). However, ensure you’re not actively using it.
5. Which is better for virtual environment management, Pipenv or venv?
Both have their pros and cons. venv
is lightweight, while Pipenv
offers a more automated approach with dependency management.
Conclusion
Python virtual environments are an invaluable tool for developers seeking clean and organized project management. By isolating dependencies, reducing conflicts, and making environments reproducible, virtual environments provide a streamlined development experience. Whether you choose venv
, Pipenv
, Conda
, or another tool, virtual environments help maintain control over project dependencies and make collaboration more effective.