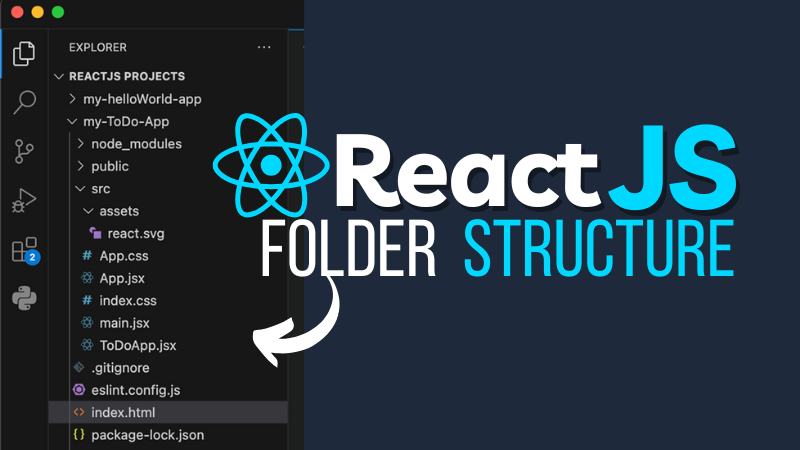
Learn how to understand ReactJS folder structure quickly with this beginner-friendly guide. Breaking down the ReactJS folder structure step-by-step for better project organization and scalability.
Introduction
ReactJS is a popular JavaScript library for building user interfaces, especially for single-page applications. While many developers focus on learning its syntax and components, understanding the ReactJS folder structure is equally important. A well-organized folder structure simplifies development, makes your code maintainable, and scales better for larger projects.
In this guide, we’ll break down the ReactJS folder structure step-by-step, show real-world examples, and provide best practices to help you master the basics quickly.
Table of Contents
Why Understanding ReactJS Folder Structure Matters
A good folder structure is the backbone of any ReactJS application. Without it, you may encounter problems like:
- Difficulty in locating files: With messy or unclear structures, finding components or assets becomes challenging.
- Scalability issues: Poor folder organization may not accommodate app growth.
- Team collaboration challenges: Clean structures promote better understanding and collaboration among team members.
Advantages of a Well-Defined Folder Structure
- Easy navigation: Quickly locate files and debug issues.
- Scalability: Add new features without reorganizing everything.
- Consistency: Follow industry-standard practices for better maintainability.
The Default ReactJS Folder Structure
When you create a React app using tools like create-react-app
or a custom setup, you’ll typically see a folder structure like this:
public/
index.html
src/
main.jsx
App.jsx
Let’s break this down:
public/
Contains static files that will not change during runtime, like:
index.html
: The main HTML file where your React app is mounted.- Images, icons, or other assets.
src/
The heart of your React app, where you write all your code. It includes:
main.jsx
: The entry point for the application.App.jsx
: The main React component.
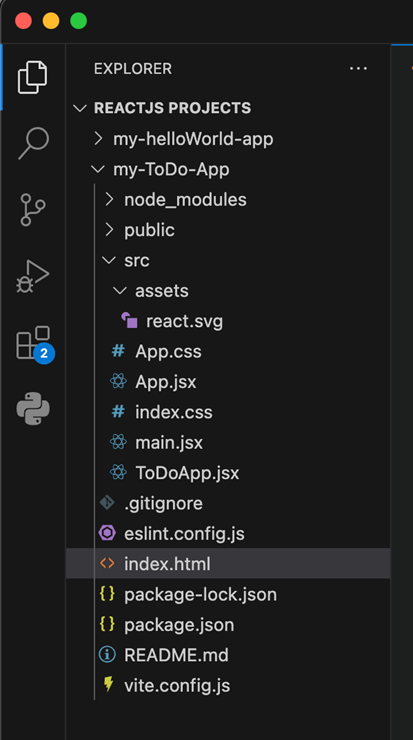
Optimizing the ReactJS Folder Structure
While the default structure is great for small projects, real-world applications often require a more modular and scalable organization. Here’s an optimized folder structure:
public/
index.html
src/
components/
Header.jsx
Footer.jsx
pages/
Home.jsx
About.jsx
styles/
App.css
utils/
helpers.js
App.jsx
main.jsx
Folder Structure Breakdown
1. components/
Holds reusable components like buttons, headers, and footers.
- Example: A
Header.jsx
component might look like this:
import React from 'react';
const Header = () => {
return (
<header>
<h1>Welcome to React</h1>
</header>
);
};
export default Header;
2. pages/
Contains specific pages of your app, like Home
or About
.
import React from 'react';
const Home = () => {
return (
<div>
<h2>Home Page</h2>
</div>
);
};
export default Home;
3. styles/
Organizes CSS files for consistent styling.
- Example: A
styles/App.css
file could include global styles:
body {
font-family: Arial, sans-serif;
}
4. utils/
Includes helper functions or services that support the app’s logic.
export const formatDate = (date) => {
return new Date(date).toLocaleDateString();
};
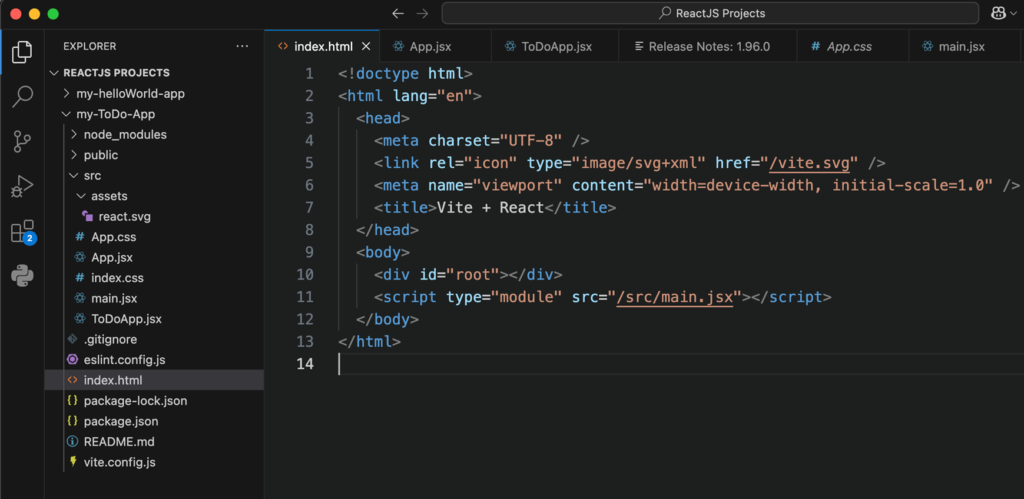
Step-by-Step Guide to Building a ReactJS App with This Structure
Let’s build a simple app with this optimized folder structure.
Real-World Problem 1: Creating a Header and Footer
Problem
We need a reusable header and footer for our website.
Solution Code
- Create
Header.jsx
andFooter.jsx
in thecomponents/
folder. - Import and use them in
App.jsx
.
Header.jsx
import React from 'react';
const Header = () => {
return <header>React App Header</header>;
};
export default Header;
Footer.jsx
import React from 'react';
const Footer = () => {
return <footer>React App Footer</footer>;
};
export default Footer;
App.jsx
import React from 'react';
import Header from './components/Header';
import Footer from './components/Footer';
const App = () => {
return (
<div>
<Header />
<main>
<p>Welcome to React!</p>
</main>
<Footer />
</div>
);
};
export default App;
Output
A page with a header, main content, and footer displayed properly.
Real-World Problem 2: Navigating Between Pages
Problem
We need a navigation system to move between Home
and About
pages.
Solution Code
Use React Router
for navigation.
- Install React Router:
npm install react-router-dom
- Update
App.jsx
:
import React from 'react';
import { BrowserRouter as Router, Route, Routes } from 'react-router-dom';
import Home from './pages/Home';
import About from './pages/About';
const App = () => {
return (
<Router>
<Routes>
<Route path="/" element={<Home />} />
<Route path="/about" element={<About />} />
</Routes>
</Router>
);
};
export default App;
Output
Users can navigate between pages using URLs /
and /about
.
Best Practices for ReactJS Folder Structure
- Keep it modular: Organize files by feature or module.
- Use meaningful names: Avoid generic names like
test.js
ornew.js
. - Separate concerns: Keep logic, styling, and components in separate folders.
- Use index.js exports: Simplifies imports for multi-file folders.
FAQs
1. Why is the public/
folder important?
The public/
folder contains static assets like index.html
, which is the entry point for your React app.
2. Should I always follow the same folder structure?
No, you can adapt the folder structure based on your project’s size and complexity.
3. What’s the difference between components/
and pages/
?
components/
: Reusable UI pieces (e.g., buttons, headers).pages/
: Full views or pages (e.g., Home, About).
4. How do I handle large-scale applications?
Consider using advanced patterns like feature-based folder structures or monorepos for better scalability.
Conclusion
Understanding the ReactJS folder structure is a key step in building efficient and scalable applications. A well-structured app simplifies collaboration, improves maintainability, and ensures scalability. By following this guide, you can quickly set up an organized React project and start developing with confidence.
Now it’s your turn—get coding and build something amazing with React!