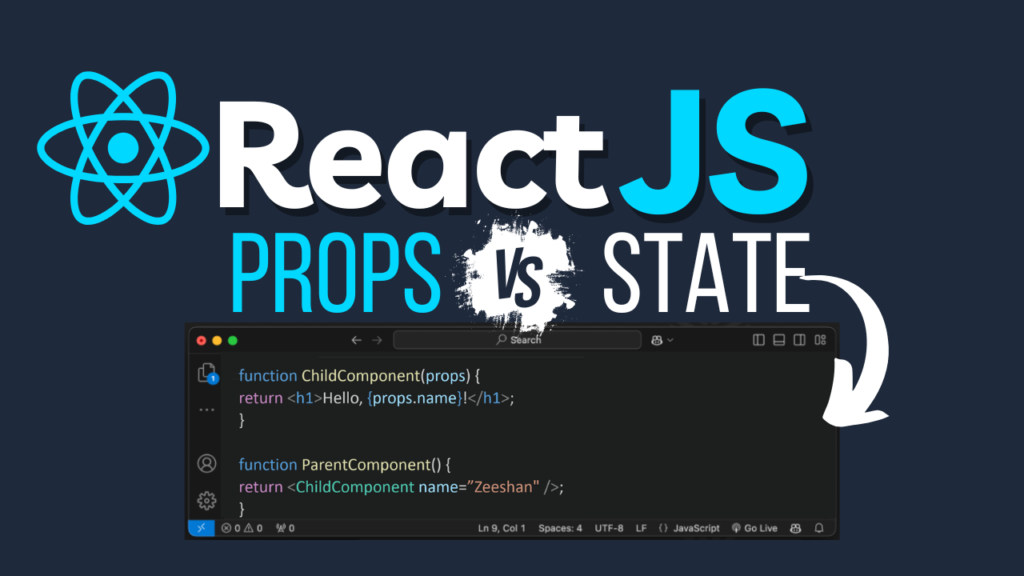
Discover the key differences between React JS Props and State. Learn when to use each, along with real-world examples, complete with code, explanations, and outputs.
Introduction to React JS Props vs State
React JS, a widely-used library for building user interfaces, offers two essential tools for managing data in components: Props and State. These two concepts often confuse beginners. However, understanding their differences and when to use each is vital for creating efficient and scalable React applications.
In this blog, we’ll explore React JS Props vs State, dive into their unique features, and illustrate their uses with six real-world examples. Whether you’re just starting with React or need a refresher, this guide will simplify these core concepts.
Table of Contents
What Are Props in React?
Props, short for properties, are a way to pass data from one component to another in React. They are read-only, meaning they cannot be modified once passed to a component. Props are ideal for making components reusable and customizable.
Key Features of Props:
- Unidirectional Data Flow: Props flow from parent to child components.
- Read-Only: They cannot be changed by the component receiving them.
- Reusable Components: Enable the creation of flexible and reusable UI components.
What Is State in React?
State is a way to manage data within a component. Unlike Props, State is mutable, meaning it can be updated by the component to reflect changes in the application. State is commonly used to handle user interactions and dynamic behavior.
Key Features of State:
- Local Data Storage: Exists only within the component that defines it.
- Dynamic Updates: Allows components to respond to user actions or system events.
- Control Over Behavior: Helps manage UI changes over time.
Differences Between Props and State
Feature | Props | State |
---|---|---|
Mutability | Immutable (read-only) | Mutable (can change) |
Scope | Passed from parent to child components | Local to the component |
Use Case | For passing data to child components | For managing dynamic data |
Updates | Cannot be updated by child components | Can be updated using setState or useState |
Real-World Examples of Props and State
Example 1: Greeting Component (Using Props)
Problem: Create a reusable Greeting component that displays a user’s name passed as a Prop.
Solution Code:
function Greeting({ name }) {
return <h1>Hello, {name}!</h1>;
}
function App() {
return (
<div>
<Greeting name="Alice" />
<Greeting name="Bob" />
</div>
);
}
Output:
- Hello, Alice!
- Hello, Bob!
Explanation:
Props allow the Greeting
component to display personalized messages for each user. This makes the component reusable and efficient.
Example 2: Counter Component (Using State)
Problem: Build a counter that increments by 1 each time a button is clicked.
Solution Code:
import { useState } from "react";
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<h1>Count: {count}</h1>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
Output:
Clicking the button updates the displayed count dynamically.
Explanation:
State is used to keep track of the counter value, and setState
updates it whenever the button is clicked.
Example 3: Temperature Display (Props and State)
Problem: Build a weather component that shows the current temperature (State) and the city name (Props).
Solution Code:
function Weather({ city }) {
const [temperature, setTemperature] = useState(25);
return (
<div>
<h1>Weather in {city}</h1>
<p>Temperature: {temperature}°C</p>
</div>
);
}
function App() {
return <Weather city="London" />;
}
Output:
- Weather in London
- Temperature: 25°C
Explanation:
Props specify the city name, while State handles the temperature, which could be updated dynamically.
Example 4: Customizable Button (Using Props)
Problem: Create a Button component that adapts its label and style based on Props.
Solution Code:
function Button({ label, style }) {
return <button style={style}>{label}</button>;
}
function App() {
return (
<div>
<Button label="Submit" style={{ backgroundColor: "blue", color: "white" }} />
<Button label="Cancel" style={{ backgroundColor: "red", color: "white" }} />
</div>
);
}
Output:
Two buttons: one labeled “Submit” and one labeled “Cancel,” each styled differently.
Explanation:
Props make the Button
component reusable by passing custom labels and styles.
Example 5: Light/Dark Mode Toggle (Using State)
Problem: Build a toggle button to switch between light and dark modes.
Solution Code:
import { useState } from "react";
function ThemeToggle() {
const [isDarkMode, setIsDarkMode] = useState(false);
return (
<div style={{
backgroundColor: isDarkMode ? "black" : "white",
color: isDarkMode ? "white" : "black",
height: "100vh",
textAlign: "center"
}}>
<h1>{isDarkMode ? "Dark Mode" : "Light Mode"}</h1>
<button onClick={() => setIsDarkMode(!isDarkMode)}>
Switch to {isDarkMode ? "Light" : "Dark"} Mode
</button>
</div>
);
}
Output:
Switching the mode updates the background and text colors dynamically.
Explanation:
State keeps track of the current mode and updates it when the button is clicked.
Example 6: Shopping Cart (Combining Props and State)
Problem: Create a shopping cart where users can add items and see the total price dynamically.
Solution Code:
function CartItem({ name, price }) {
return (
<div>
<h2>{name}</h2>
<p>Price: ${price}</p>
</div>
);
}
function ShoppingCart() {
const [cart, setCart] = useState([]);
const [total, setTotal] = useState(0);
const addItem = (item) => {
setCart([...cart, item]);
setTotal(total + item.price);
};
return (
<div>
<button onClick={() => addItem({ name: "Item 1", price: 10 })}>Add Item 1 ($10)</button>
<button onClick={() => addItem({ name: "Item 2", price: 20 })}>Add Item 2 ($20)</button>
<h1>Shopping Cart</h1>
{cart.map((item, index) => (
<CartItem key={index} name={item.name} price={item.price} />
))}
<h2>Total: ${total}</h2>
</div>
);
}
Output:
Adding items updates the cart list and total dynamically.
Explanation:
Props display individual item details, while State tracks the cart contents and total price.
FAQs
1. What is the main difference between Props and State in React?
Props are immutable and passed from parent to child components. State is mutable and managed within the component.
2. When should I use Props in React?
Use Props for passing data and configuration from a parent to child components.
3. How do I update State in React?
Use setState
in class components or the useState
hook in functional components to update State.
Conclusion
Understanding React JS Props vs State is crucial for building efficient React applications. By leveraging Props for customization and State for dynamic updates, you can create scalable and responsive user interfaces. Use these real-world examples to practice and master these concepts in your projects.