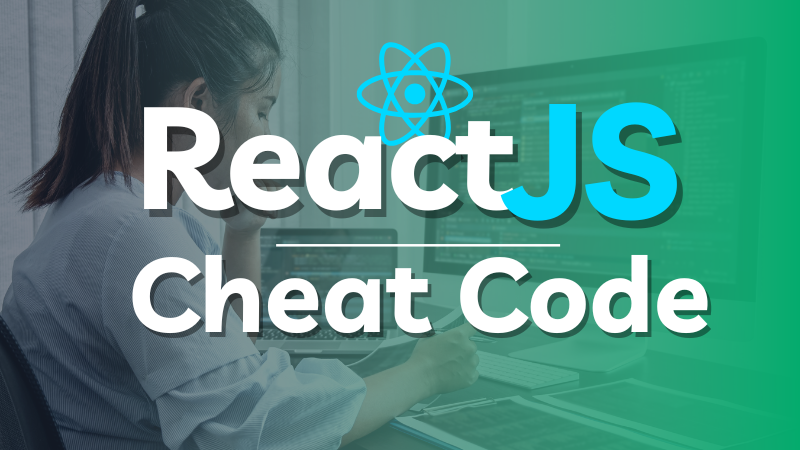
Learn React JS using this quick and easy to follow short course. This direct source code based cheat sheet will take you through all major concepts of ReactJS and give you examples of how ReactJS code works.
1. ReactJS Introduction
- React is a JavaScript library for building user interfaces.
- React is used to build single-page applications.
- React allows us to create reusable UI components.
- For more information, visit https://reactjs.org/
2. ReactJS basic Syntax
import React from 'react';
import ReactDOM from 'react-dom';
// Simple React component
function App() {
return <h1>Hello, React!</h1>;
}
ReactDOM.render(<App />, document.getElementById('root'));
3. ReactJS Get Started
To use React in production, you need npm which is included with Node.js(https://nodejs.org/).
Setting up a React Environment
If you have npx and Node.js installed, you can create a React application by using create-react-app.
// Use Create React App to start a new project:
> npx create-react-app my-appName
//Run this command to move to the my-react-app directory:
> cd my-appName
//Run this command to run the React application my-appName:
> npm start
4. React Upgrade
// To upgrade React:
> npm install react@latest react-dom@latest
5. React ES6 Arrow Function
const App = () => {
const [name, setName] = React.useState('React');
return <h1>Hello, {name}!</h1>;
};
6. React Render HTML
function Welcome() {
return (
<div>
<h1>Welcome to React!</h1>
<p>This is a simple component.</p>
</div>
);
}
ReactDOM.render(<Welcome />, document.getElementById('root'));
7. React JSX
const element = <h1>Hello, JSX!</h1>;
ReactDOM.render(element, document.getElementById('root'));
8. React Components
// Functional component
function MyComponent() {
return <div>This is a functional component</div>;
}
// Class component
class MyClassComponent extends React.Component {
render() {
return <div>This is a class component</div>;
}
9. React Class
class Counter extends React.Component {
constructor(props) {
super(props);
this.state = { count: 0 };
}
increment = () => {
this.setState({ count: this.state.count + 1 });
};
render() {
return (
<div>
<p>Count: {this.state.count}</p>
<button onClick={this.increment}>Increment</button>
</div>
);
}
}
10. React Props
function Greeting(props) {
return <h1>Hello, {props.name}!</h1>;
}
ReactDOM.render(<Greeting name="World" />, document.getElementById('root'));
11. React Events
function App() {
const handleClick = () => {
alert('Button clicked!');
};
return <button onClick={handleClick}>Click me</button>;
}
12. React Conditionals
function Welcome(props) {
if (props.isLoggedIn) {
return <h1>Welcome back!</h1>;
} else {
return <h1>Please sign up.</h1>;
}
}
13. React Lists
const items = ['Item 1', 'Item 2', 'Item 3'];
function ItemList() {
return (
<ul>
{items.map((item, index) => (
<li key={index}>{item}</li>
))}
</ul>
);
}
14. React Forms
function MyForm() {
const [value, setValue] = React.useState('');
const handleChange = (event) => {
setValue(event.target.value);
};
const handleSubmit = (event) => {
alert('A name was submitted: ' + value);
event.preventDefault();
};
return (
<form onSubmit={handleSubmit}>
<label>
Name:
<input type="text" value={value} onChange={handleChange} />
</label>
<button type="submit">Submit</button>
</form>
);
}
15. React Router
import { BrowserRouter as Router, Route, Switch } from 'react-router-dom';
function Home() {
return <h2>Home</h2>;
}
function About() {
return <h2>About</h2>;
}
function App() {
return (
<Router>
<div>
<nav>
<ul>
<li><a href="/">Home</a></li>
<li><a href="/about">About</a></li>
</ul>
</nav>
<Switch>
<Route path="/" exact component={Home} />
<Route path="/about" component={About} />
</Switch>
</div>
</Router>
);
}
16. React Memo
const ExpensiveComponent = React.memo(function ExpensiveComponent({ data }) {
// Expensive calculation here
return <div>{data}</div>;
});
17. React CSS Styling
function StyledComponent() {
const style = { color: 'blue', fontSize: '20px' };
return <div style={style}>Styled Text</div>;
}
18. React Sass Styling
// styles.scss
/*
$primary-color: #333;
.container {
color: $primary-color;
padding: 20px;
}
*/
// In your component file
import './styles.scss';
function StyledComponent() {
return <div className="container">Sass Styled Text</div>;
}
19. React Hooks: What is a Hook?
Hooks are functions that let you use state and other React features without writing a class.
20. React Hooks: useState
function Counter() {
const [count, setCount] = React.useState(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
21. React Hooks: useEffect
function Example() {
React.useEffect(() => {
document.title = 'React Hook Example';
});
return <div>Check the document title</div>;
}
22. React Hooks: useContext
const ThemeContext = React.createContext('light');
function ThemedComponent() {
const theme = React.useContext(ThemeContext);
return <div>Current theme: {theme}</div>;
}
23. React Hooks: useRef
function FocusInput() {
const inputRef = React.useRef(null);
const focusInput = () => {
inputRef.current.focus();
};
return (
<div>
<input ref={inputRef} type="text" />
<button onClick={focusInput}>Focus Input</button>
</div>
);
}
24. React Hooks: useReducer
function reducer(state, action) {
switch (action.type) {
case 'increment':
return { count: state.count + 1 };
case 'decrement':
return { count: state.count - 1 };
default:
throw new Error();
}
}
function Counter() {
const [state, dispatch] = React.useReducer(reducer, { count: 0 });
return (
<div>
Count: {state.count}
<button onClick={() => dispatch({ type: 'increment' })}>Increment</button>
<button onClick={() => dispatch({ type: 'decrement' })}>Decrement</button>
</div>
);
}
25. React Hooks: useCallback
function List({ items, onItemClick }) {
const handleClick = React.useCallback((item) => {
onItemClick(item);
}, [onItemClick]);
return (
<ul>
{items.map(item => (
<li key={item} onClick={() => handleClick(item)}>
{item}
</li>
))}
</ul>
);
}
26. React Hooks: useMemo
function ExpensiveComponent({ data }) {
const calculatedValue = React.useMemo(() => {
// Expensive calculation
return data.reduce((sum, value) => sum + value, 0);
}, [data]);
return <div>Calculated Value: {calculatedValue}</div>;
}