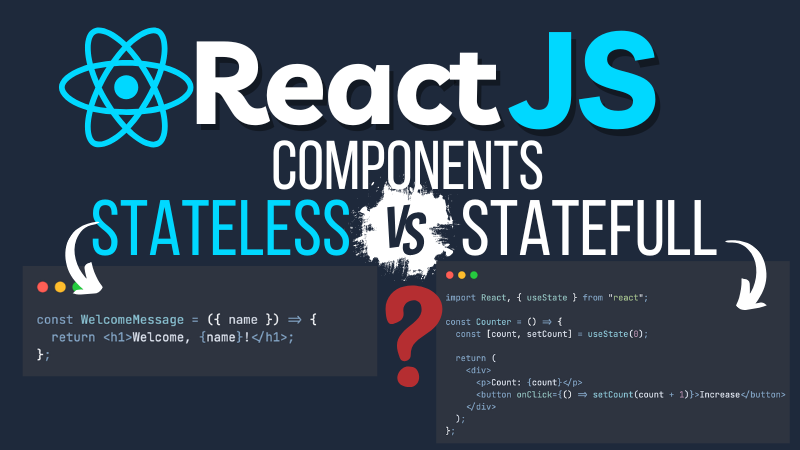
Learn the differences between Stateless and Stateful Components in React with real-world examples, detailed explanations, and source code solutions. A beginner-friendly guide to mastering React components and data flow. Clear your confusion about ReactJS components with this comprehensive guide on Stateless vs. Stateful Components. Learn with detailed explanations, examples, and best practices to make your React development seamless.
Introduction
ReactJS is a powerful library for building user interfaces, but many beginners face confusion when it comes to understanding its components. The concepts of Stateless and Stateful Components are fundamental in React, yet distinguishing between them can be tricky at first.
This blog aims to clarify the differences, usage, and benefits of these two types of components. We’ll dive deep into their functionalities, explore examples, and show you how they work together to create dynamic and efficient applications. Whether you’re a novice or looking to refine your knowledge, this guide will provide value and actionable insights.
Table of Contents
What Are ReactJS Components?
The Building Blocks of ReactJS
ReactJS components are the essential building blocks of any React application. They allow developers to create reusable and modular pieces of UI. Think of components as the LEGO bricks of a React app: they can be stacked together to build complex interfaces while being individually manageable.
Components can either be Stateless or Stateful, depending on their role in managing data and interactions.
How Do Components Work in ReactJS?
ReactJS components follow a simple yet powerful pattern:
- Input: Components accept inputs called props (short for properties).
- Processing: They may or may not manage their own internal data (state).
- Output: They return a UI representation, usually in the form of JSX (JavaScript XML).
Code Example: Basic React Component
Here’s an example of a simple React component that renders a greeting message:
const Greeting = () => {
return <h1>Hello, ReactJS!</h1>;
};
This example demonstrates how a stateless functional component can display static content.
Stateless Components: The Presentational Heroes
What Are Stateless Components?
Stateless components, also known as functional components, are those that do not manage their own state. They are solely responsible for rendering UI based on the props they receive. They are ideal for creating reusable, presentation-focused pieces of your application.
Advantages of Stateless Components
- Simplicity: They have a clear focus on rendering and are easy to read.
- Reusability: You can use them across your application with different data via props.
- Performance: They don’t have any overhead related to state management, making them faster.
Example Code: Stateless Component
const WelcomeMessage = ({ name }) => {
return <h1>Welcome, {name}!</h1>;
};
In this example, the
WelcomeMessage
component accepts aname
prop and renders a personalized greeting.
When to Use Stateless Components
Stateless components are perfect for creating elements like:
- Navigation bars
- Buttons
- Headings and footers
Stateful Components: Managing Data Dynamically
What Are Stateful Components?
Stateful components are those that manage their own internal state. They are often used for dynamic and interactive UI elements. Unlike stateless components, they are typically created using class components or React hooks like useState
.
Advantages of Stateful Components
- Dynamic Behavior: They can update their UI based on internal state changes.
- Flexibility: Ideal for handling user inputs and interactions.
- Self-Contained: They encapsulate their own logic, making them powerful and versatile.
Example Code: Stateful Component
import React, { useState } from "react";
const Counter = () => {
const [count, setCount] = useState(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increase</button>
</div>
);
};
In this example, the
Counter
component maintains its own state (count
) and provides functionality to increment it.
When to Use Stateful Components
Stateful components are best suited for scenarios like:
- Managing forms
- Handling user interactions (e.g., buttons, toggles)
- Implementing dynamic data fetching
Stateless vs. Stateful Components: A Practical Comparison
Understanding Their Differences
Feature | Stateless Components | Stateful Components |
---|---|---|
State Management | No | Yes |
Use Case | Static UI | Dynamic and interactive UI |
Complexity | Low | Medium to High |
Performance | High | Slightly lower |
Examples | Navbar, Buttons, Cards | Forms, Modals, Toggles |
Code Examples and Use Cases
Example 1: Shopping List
Let’s create a shopping list app where users can dynamically add items to their list.
Stateless Component: Item
const Item = ({ name }) => <li>{name}</li>;
Stateful Component: ShoppingList
const ShoppingList = () => {
const [items, setItems] = React.useState(["Milk", "Eggs"]);
const addItem = () => setItems([...items, "Bread"]);
return (
<div>
<ul>
{items.map((item, index) => (
<Item key={index} name={item} />
))}
</ul>
<button onClick={addItem}>Add Bread</button>
</div>
);
};
Output
- Initial State: Displays “Milk” and “Eggs.”
- After Interaction: Adds “Bread” to the list dynamically.
Example 2: User Profile Editor
Stateless Component: UserDetails
const UserDetails = ({ name, age }) => {
return (
<div>
<h2>{name}</h2>
<p>Age: {age}</p>
</div>
);
};
Stateful Component: UserProfile
onst UserProfile = () => {
const [name, setName] = React.useState("Alice");
const [age, setAge] = React.useState(25);
return (
<div>
<UserDetails name={name} age={age} />
<input
type="text"
value={name}
onChange={(e) => setName(e.target.value)}
/>
<button onClick={() => setAge(age + 1)}>Increase Age</button>
</div>
);
};
Output
Allows live editing of the user’s name and increases their age dynamically.
Key Takeaways
- Stateless Components: Ideal for static UI and reusable elements.
- Stateful Components: Essential for managing dynamic, interactive elements.
- Integration: Combine both types for efficient, scalable applications.
FAQs
1. What Are ReactJS Components?
ReactJS components are the building blocks of any React application. They allow developers to create modular, reusable UI elements.
2. What Is the Main Difference Between Stateless and Stateful Components?
Stateless components do not manage internal state and focus on rendering, while stateful components manage internal state and handle user interactions.
3. Can Stateless and Stateful Components Be Used Together?
Yes! They often work together to build a cohesive application. For example, a stateful component can manage data and pass it to stateless components for rendering.
Conclusion
By understanding the differences between Stateless and Stateful Components, you can create more efficient and maintainable React applications. Use this guide to master ReactJS components and clear any confusion once and for all. With practice, you’ll build apps that are dynamic, scalable, and user-friendly.