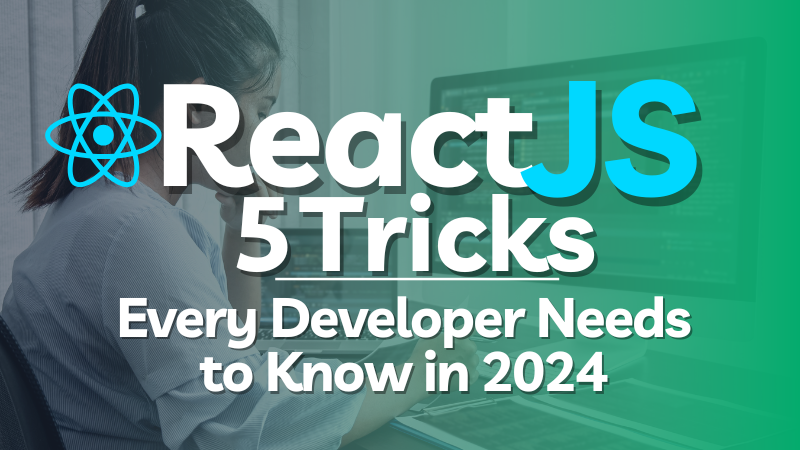
Discover the top 5 essential React JS tricks every developer should master in 2024. From hooks to performance optimization, enhance your React development with these powerful techniques.
Introduction
React JS has taken the web development world by storm, and with good reason. Its component-based architecture, coupled with an active community, has made it one of the most popular libraries for building user interfaces. However, even seasoned React developers can benefit from learning some new tricks to make their development process faster, cleaner, and more efficient. In this article, we will explore 5 essential React JS tricks every developer should know in 2024. Whether you’re a beginner or a seasoned pro, these tips will enhance your workflow, help optimize your code, and boost your productivity.
Let’s dive into these five tricks that can improve your React development game.
H1: 1. Mastering React Hooks for Cleaner and More Efficient Code
React hooks are one of the most powerful features introduced in React 16.8. They allow you to use state and lifecycle features in functional components, which previously could only be done in class components. Hooks simplify the way we write React components and help improve code readability.
H2: Why Hooks are Essential in ReactJS
Before hooks, state management and lifecycle methods like componentDidMount()
were limited to class components. With hooks, you can use the same functionality in functional components, making your code cleaner and easier to understand.
H3: Basic Hooks Examples
- useState:
This hook allows you to add state to your functional components. Let’s see how it wo
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
Here, useState
initializes the count
variable with a value of 0
and provides a function (setCount
) to update it.
2. useEffect:
The useEffect
hook is used to perform side effects in your components. This can include fetching data, manually changing the DOM, or setting up subscriptions.
import React, { useState, useEffect } from 'react';
function FetchData() {
const [data, setData] = useState(null);
useEffect(() => {
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => setData(data));
}, []); // Empty array means it only runs once when the component mounts
return <div>{data ? JSON.stringify(data) : "Loading..."}</div>;
}
3. useContext:
This hook allows you to use context in functional components. It simplifies passing data through the component tree without the need for props drilling.
import React, { createContext, useContext } from 'react';
const ThemeContext = createContext('light');
function ThemedComponent() {
const theme = useContext(ThemeContext);
return <div>{theme === 'dark' ? 'Dark Mode' : 'Light Mode'}</div>;
}
function App() {
return (
<ThemeContext.Provider value="dark">
<ThemedComponent />
</ThemeContext.Provider>
);
}
H3: Advanced Hook Usage for Optimization in ReactJS
Beyond the basics, hooks like useMemo
, useCallback
, and useReducer
can be used to optimize performance in larger applications. These advanced hooks can help you prevent unnecessary renders, memoize values, and manage complex state logic.
H1: 2. Performance Optimization with React.memo and useMemo in ReactJS
React’s React.memo()
and useMemo()
are performance optimization techniques that can help make your application faster and more efficient. Let’s explore how each of them works and why you should use them.
H2: Using React.memo for Component Optimization
React.memo()
is a higher-order component that prevents unnecessary re-renders of functional components. It only re-renders the component if its props change, which can drastically improve performance, especially for large apps.
import React, { memo } from 'react';
const ListItem = memo(({ item }) => {
console.log('Rendering:', item.name);
return <div>{item.name}</div>;
});
function List() {
const items = [{ name: 'Item 1' }, { name: 'Item 2' }];
return (
<div>
{items.map(item => (
<ListItem key={item.name} item={item} />
))}
</div>
);
}
In the above example,
ListItem
will only re-render if theitem
prop changes.
H3: Using useMemo for Expensive Calculations
useMemo()
is a hook that helps memoize expensive calculations. This ensures that the calculation is only recomputed when the dependencies change, rather than on every render.
import React, { useMemo } from 'react';
function ExpensiveComputation({ number }) {
const factorial = useMemo(() => {
let result = 1;
for (let i = 1; i <= number; i++) {
result *= i;
}
return result;
}, [number]);
return <div>Factorial: {factorial}</div>;
}
Here, the factorial calculation will only be recomputed if the
number
prop changes.
H1: 3. Leveraging Code-Splitting for Faster Load Times in ReactJS
Code-splitting is a technique that allows you to split your bundle into smaller chunks, which are only loaded when needed. This can significantly improve the performance and user experience of your React app.
H2: What is Code-Splitting?
Code-splitting is a technique to break your JavaScript bundle into smaller pieces. This way, only the necessary parts of your app are loaded initially, and the rest can be loaded asynchronously as needed.
H3: React Lazy and Suspense for Code-Splitting
React provides built-in tools for code-splitting: React.lazy()
and Suspense
.
React.lazy()
is used to dynamically import components.Suspense
is used to handle loading states when the component is being loaded asynchronously.
import React, { Suspense, lazy } from 'react';
const LazyComponent = lazy(() => import('./LazyComponent'));
function App() {
return (
<Suspense fallback={<div>Loading...</div>}>
<LazyComponent />
</Suspense>
);
}
In the example above,
LazyComponent
will only be loaded when it’s needed, which reduces the initial bundle size.
H1: 4. Understanding and Using Error Boundaries in ReactJS
Error Boundaries are a powerful feature in React that allow you to catch JavaScript errors anywhere in your component tree, log those errors, and display a fallback UI. They are particularly useful in large applications where errors can happen in unpredictable ways.
H2: What Are Error Boundaries?
An Error Boundary is a higher-order component that catches errors in its child components and provides a fallback UI instead of crashing the whole app.
H3: Creating an Error Boundary Component
Here’s an example of how to create an error boundary:
import React, { Component } from 'react';
class ErrorBoundary extends Component {
constructor(props) {
super(props);
this.state = { hasError: false };
}
static getDerivedStateFromError(error) {
return { hasError: true };
}
componentDidCatch(error, errorInfo) {
console.log(error, errorInfo);
}
render() {
if (this.state.hasError) {
return <h1>Something went wrong.</h1>;
}
return this.props.children;
}
}
export default ErrorBoundary;
You can then wrap your components with
ErrorBoundary
to catch errors in any of their children.
function App() {
return (
<ErrorBoundary>
<ComponentThatMightThrow />
</ErrorBoundary>
);
}
H1: 5. Using PropTypes for Type Checking in ReactJS
PropTypes is a built-in feature in React that helps developers ensure the correct type of props are passed to components. By enforcing type checking, you can avoid bugs and enhance the maintainability of your code.
H2: What Are PropTypes?
PropTypes is a way to validate the types of props your components receive. This helps catch bugs early and improve code quality.
H3: Adding PropTypes to Your Components
Here’s how to use PropTypes to validate the types of your component props:
import PropTypes from 'prop-types';
function UserProfile({ name, age }) {
return (
<div>
<h1>{name}</h1>
<p>{age}</p>
</div>
);
}
UserProfile.propTypes = {
name: PropTypes.string.isRequired,
age: PropTypes.number.isRequired,
};
H2: 6. Bonus Tip: Leveraging Custom Hooks for Reusable Logic
Custom hooks are a way to extract and reuse logic in your React applications. They allow you to encapsulate reusable behavior and make your components cleaner and easier to understand.
H3: Why Use Custom Hooks?
Custom hooks enable developers to share stateful logic across multiple components without duplicating code. They help make your codebase DRY (Don’t Repeat Yourself) and modular.
H3: Creating a Custom Hook
Let’s create a custom hook to fetch data from an API. This is a common task that can benefit from abstraction.
import { useState, useEffect } from 'react';
function useFetch(url) {
const [data, setData] = useState(null);
const [loading, setLoading] = useState(true);
useEffect(() => {
fetch(url)
.then(response => response.json())
.then(data => {
setData(data);
setLoading(false);
});
}, [url]);
return { data, loading };
}
You can use the useFetch
hook in multiple components without rewriting the fetching logic:
function UsersList() {
const { data, loading } = useFetch('https://api.example.com/users');
if (loading) return <p>Loading...</p>;
return (
<ul>
{data.map(user => (
<li key={user.id}>{user.name}</li>
))}
</ul>
);
}
H3: Custom Hooks for Form Handling
Here’s another example: a custom hook for form state management.
import { useState } from 'react';
function useForm(initialValues) {
const [values, setValues] = useState(initialValues);
const handleChange = (event) => {
const { name, value } = event.target;
setValues({
...values,
[name]: value,
});
};
return { values, handleChange };
}
Usage:
function LoginForm() {
const { values, handleChange } = useForm({ username: '', password: '' });
const handleSubmit = (event) => {
event.preventDefault();
console.log(values);
};
return (
<form onSubmit={handleSubmit}>
<input
name="username"
value={values.username}
onChange={handleChange}
placeholder="Username"
/>
<input
name="password"
value={values.password}
onChange={handleChange}
placeholder="Password"
type="password"
/>
<button type="submit">Login</button>
</form>
);
}
By encapsulating form logic in
useForm
, you can easily reuse it across multiple components.
H2: Best Practices for React Developers in 2024
As React continues to evolve, adhering to best practices ensures your code is future-proof, maintainable, and performant. Here are some additional tips:
- Use TypeScript with React:
TypeScript enhances your codebase by catching type errors during development, making it more robust and easier to debug. - Adopt Component Libraries:
Leverage libraries like Material-UI or Ant Design for faster UI development and consistent design patterns. - Test Your Components:
Use tools like Jest and React Testing Library to write tests that ensure your components work as expected. - Optimize Build Tools:
Stay up to date with build tools like Vite, Webpack, or esbuild for faster development and production builds. - Focus on Accessibility:
Make your application accessible by following WCAG guidelines and using tools like React ARIA for improved inclusivity.
H1: Key Takeaways
React JS is a versatile library, but mastering advanced tricks can significantly elevate your development skills. Here’s what we covered:
- Leveraging hooks (
useState
,useEffect
,useContext
, and more) for cleaner code. - Optimizing performance with
React.memo
,useMemo
, and code-splitting techniques. - Using error boundaries to handle unexpected errors gracefully.
- Enforcing type safety with PropTypes.
- Creating reusable custom hooks for better modularity.
- Adopting modern best practices to stay ahead in React development.
By implementing these techniques, you’ll not only improve your current projects but also prepare yourself for future React trends and challenges.
H1: FAQs (Continued)
H2: 6. How do I choose between functional and class components in React?
Functional components are now the standard choice for React development, especially with the introduction of hooks. Class components are still supported but are less commonly used in modern projects.
H2: 7. What’s the difference between useEffect
and componentDidMount
?
useEffect
is the functional component equivalent of componentDidMount
, componentDidUpdate
, and componentWillUnmount
. It allows you to perform side effects in a unified way.
H2: 8. Is it necessary to use TypeScript in React projects?
While not mandatory, TypeScript can greatly enhance your code’s reliability by adding static typing, making it easier to catch bugs early in development.
H2: 9. Can I use Redux and hooks together?
Yes, Redux works seamlessly with hooks. You can use useSelector
to access the Redux state and useDispatch
to dispatch actions, eliminating the need for higher-order components like connect
.
H2: 10. What’s new in React in 2024?
React in 2024 emphasizes improved performance, concurrent rendering with React 18 features, and better developer tools for debugging and optimizing your applications.
Conclusion
React JS continues to be a leader in web development, and staying updated with the latest techniques is crucial for any developer. By incorporating these 5 React tricks into your workflow, you’ll not only write cleaner, more efficient code but also stay ahead in the rapidly evolving world of front-end development.
Experiment with these tips in your projects, and don’t forget to share your success stories. Let’s build better React apps in 2024!